Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial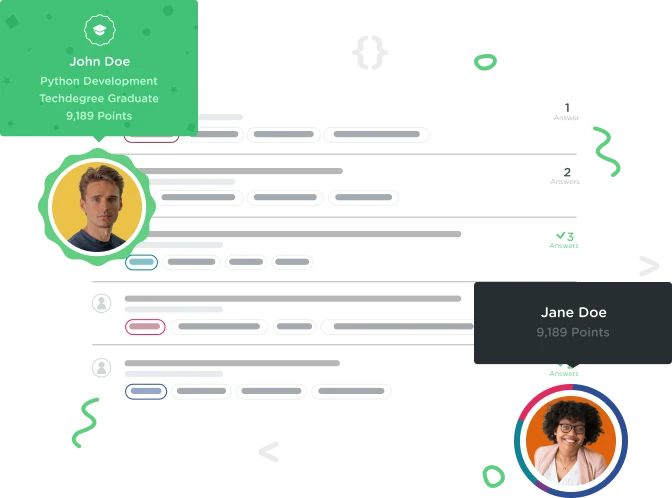
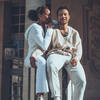
Maximilian Hill
Python Development Techdegree Graduate 7,661 PointsUnit Project 4: Wrong number of product Id's
I am reading the CSV file using dict reader, but I am getting the wrong number of product Id's, 83 instead of 26. I am assuming it's somewhere in my add_csv function, probably a loop, but I need help with it. Can anyone let me know where I am making the mistake.
Here is my app file:
from models import (Base, session, Product, engine)
import datetime
import csv
import time
def menu():
choice =input('''
\n***** Main Menu *****
\rPlease make a selection
\rPress 'v' View details
\rPress 'a' to add item
\rpress 'b' to make a back up of all contets
\rPress 'e' to exit
\r
\rMake your selection: ''')
if choice in ['v', 'a', 'b', 'e' ]:
return choice
def clean_date(date_str):
try:
date_object = datetime.datetime.strptime(date_str, "%m/%d/%Y")
format_date =date_object.strftime("%Y-%m-%d")
return_date = datetime.datetime.strptime(format_date, '%Y-%m-%d').date()
except ValueError:
input( '''
\n******** DATE ERROR *********
\rThe Date format should inlcude a valid Month Day, Year from the past
\rExample: January 13, 2003
\rPress ENTER to try again
\r*********************************
\r''')
return
else:
return return_date
def clean_price(price_str):
cleaned_price = float(price_str.strip("$"))
cleaned_price = float(cleaned_price)
cleaned_price = int(cleaned_price * 100)
return cleaned_price
def clean_id(id_str, options):
try:
book_id = int(id_str)
except:
input( f'''
\n******** Id ERROR *********
\rId must be a number.
\rPress ENTER to try again
\r***************************''')
return
else:
if book_id in options:
return book_id
else:
input( '''
\n******** Id ERROR *********
\r Options are: {my_options}.
\rPress ENTER to try again
\r***************************''')
def add_csv():
with open('inventory.csv') as csvfile:
data = csv.DictReader(csvfile)
new_data =[]
for row in data:
clean_rows = {}
clean_rows['product_name'] = row['product_name']
prices = row.get('product_price', 'N/A')
prices = clean_price(prices)
clean_rows['product_price'] = prices
quants = row['product_quantity']
quants = int(quants)
clean_rows['product_quantity'] = quants
dates = row.get('date_updated', 'N/A')
dates = clean_date(dates)
clean_rows['date_updated'] = dates
new_data.append(clean_rows)
for item in new_data:
product = Product(**item)
session.add(product)
session.commit
def app():
add_csv()
app_running = True
while app_running:
choice = menu()
if choice == 'v':
id_options = []
for item in session.query(Product):
id_options.append(item.product_id)
id_error = True
while id_error:
pick_id = input(f'''
\nId options = {id_options}
\rMake your selection: ''')
pick_id = clean_id(pick_id, id_options)
if type(pick_id) == int:
id_error = False
the_product = session.query(Product).filter(Product.product_id == pick_id).first()
print(f'''
\nProduct name: {the_product.product_name}
\rProduct Price: ${(the_product.product_price) / 100}
\rStock: {the_product.product_quantity}
\rStock updated on: {(the_product.date_updated)}
\r''')
input('Press ENTER to continue')
elif choice == 'a':
pass
elif choice == 'b':
pass
else:
print("Inventory Closing: Goodbye!")
app_running = False
if __name__ == "__main__":
Base.metadata.create_all(engine)
add_csv()
for item in session.query(Product):
print(item.product_id)
Here is my model file:
from sqlalchemy import (create_engine, Column,
String, Integer, Date)
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
engine = create_engine('sqlite:///inventory.db.', echo = False)
Session = sessionmaker(bind = engine)
session = Session()
Base = declarative_base()
class Product(Base):
__tablename__ = 'Products'
product_id = Column(Integer, primary_key= True)
product_name = Column('Name',String )
product_price = Column('Price', Integer)
product_quantity = Column('Quantity', Integer)
date_updated = Column('Date Updated', Date)
def __repr__(self):
return (f'Name: {self.product_name} Quantity: {self.product_quantity} Price:{self.product_price} Date Updated: {self.date_updated}')
1 Answer
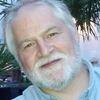
Jeff Muday
Treehouse Moderator 28,717 PointsI don't know the exact nature of your program.
Perhaps you added your data multiple times (during multiple runs) rather than starting with a "clean" database?