Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial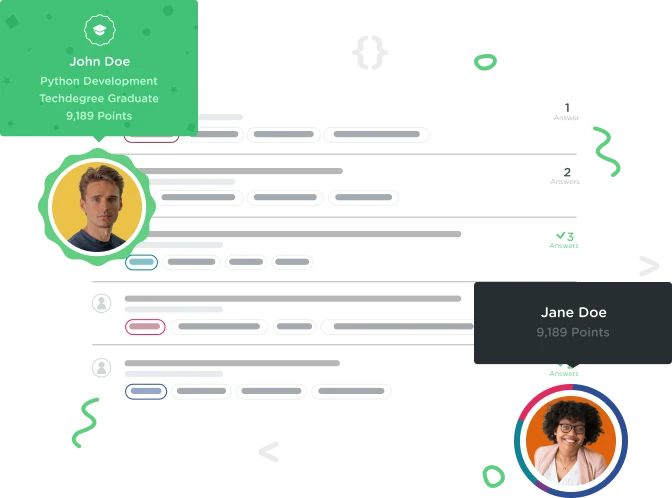

Enyang Mercy
Courses Plus Student 2,339 PointsUnit Test in java Calculator Test @BeforeMethod
How do i fix the arrange part? I already instantiated a new Calculator in each method. But i can't compile . Can u please identify the error and make corrections. Thanks
package com.example;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class CalculatorTest {
@Before
public void setUp() throws Exception {
Calculator = new Calculator();
}
@Test
public void addingMultipleNumbersProducesResult() throws Exception {
Calculator calculator = new Calculator();
int answer = calculator.addNumbers(1 ,2, 3);
assertEquals(6, answer);
}
@Test
public void addingSingleNumberTotalsAppropriately() throws Exception {
Calculator calculator = new Calculator();
int answer = calculator.addNumbers(1);
assertEquals(1, answer);
}
}
package com.example;
public class Calculator {
public int addNumbers(int... numbers) {
int total = 0;
for (int number : numbers) {
total += number;
}
return total;
}
}
1 Answer
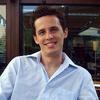
Pedro Cabral
33,586 PointsYou have a couple of problems:
- In your before method, you declared an object of type Calculator but you did not assign it a variable name.
- The point of having the Calculator in the before method is to avoid repetition in your tests, so you can remove those repeated assignments/instantiations as you are meant to initialise the calculator only once.
- Once you remove it from your test methods, you need a way to access it, so you should move calculator to a field/property of the class and leave the instantiation in your before method so that it will be available to all tests.