Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial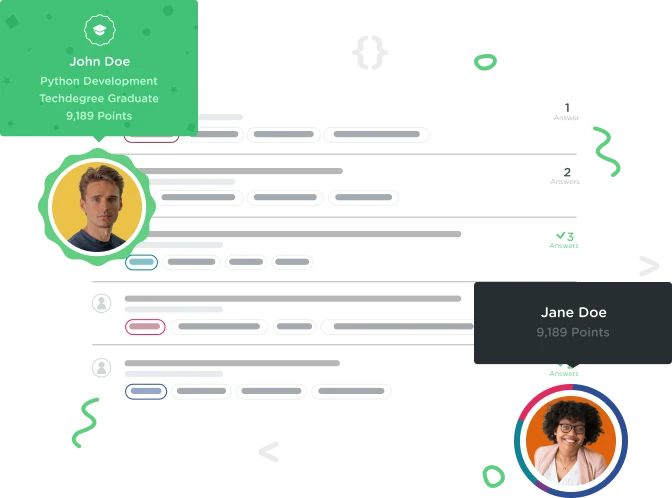

Enyang Mercy
Courses Plus Student 2,339 PointsUnit testing CreditorTest
Write a happy path test using expected element. Help!!! i can't really figure out what is required
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class CreditorTest {
private Creditor creditor;
@Before
public void setUp() throws Exception {
creditor = new Creditor();
}
@Test
public void addingFundsIncrementsAvailableFunds() throws Exception {
creditor.addFunds(25);
creditor.addFunds(25);
assertEquals(50, creditor.getAvailableFunds());
}
@Test
public void refundingReturnsAllAvailableFunds() throws Exception {
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(10, refund);
}
@Test
public void refundingResetsAvailableFundsToZero() throws Exception {
creditor.addFunds(10);
creditor.refund();
assertEquals(0, creditor.getAvailableFunds());
}
}
package com.teamtreehouse.vending;
public class Creditor {
private int funds;
public Creditor() {
funds = 0;
}
public void addFunds(int money) {
funds += money;
}
public void deduct(int money) throws NotEnoughFundsException {
if (money > funds) {
throw new NotEnoughFundsException();
}
funds -= money;
}
public int refund() {
int refund = funds;
funds = 0;
return refund;
}
public int getAvailableFunds() {
return funds;
}
}
package com.teamtreehouse.vending;
public class NotEnoughFundsException extends Exception {
}
3 Answers

Sean M
7,344 PointsThis question took me the longest in the Java course thus far. I thought I would share my answer for future reference.
- In simplest terms, you're trying to stop the vending machine from deducting more money than what's in the available funds. Therefore, it will throw an exception to prevent this from happening.
public void deductMoreMoneyThanAvailableFunds() throws Exception {
- Yanuar made a good point that every @Test method is isolated from each other. Therefore the available funds is 0. Therefore, if we try to deduct even 1 dollar or more, there will not be enough funds since $0 is available
creditor.deduct(1);
Final Code.
@Test (expected = NotEnoughFundsException.class)
public void deductMoreMoneyThanAvailableFunds() throws Exception {
creditor.deduct(1);
}
}

Yanuar Prakoso
15,196 PointsHi There
Basically you need to create a test on what will be happening if the creditor deduct money larger than available fund in the vending machine. You need to create a test scenario where the result will invoke an Error. The Error is specific called NotEnoughFundsException, which must be mentioned in the Test annotation as expected parameter. I just add this code in the CreditorTest.java:
@Test(expected = NotEnoughFundsException.class)
public void deductMoreMoneyThanAvailableFunds() throws Exception{
creditor.deduct(10);
}
You might wondering in the tests above there are few funds submitted right? They add 25 twice in the first test and then later on 10 in the later test. So if in my code I only deduct 10 dollar would it be just okay? Would it be failed to invoke the NotEnoughFundException?
The answer is no. Each of those test is Isolated like in the FIRST concept Craig talked about in the earlier video. Meaning my last @Test code has nothing to do with other @Test even it is in the same test class. Thus in my Test code the available fund in the vending machine is = 0.
Therefore, when I deduct 10 dollar from the vending machine there will not be enough fund available in the vending machine. Meaning it will invoke the NotEnoughFundException as expected.
I hope this helps a little. Happy coding
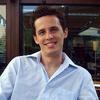
Pedro Cabral
33,586 PointsWhy create a second thread about the same?