Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial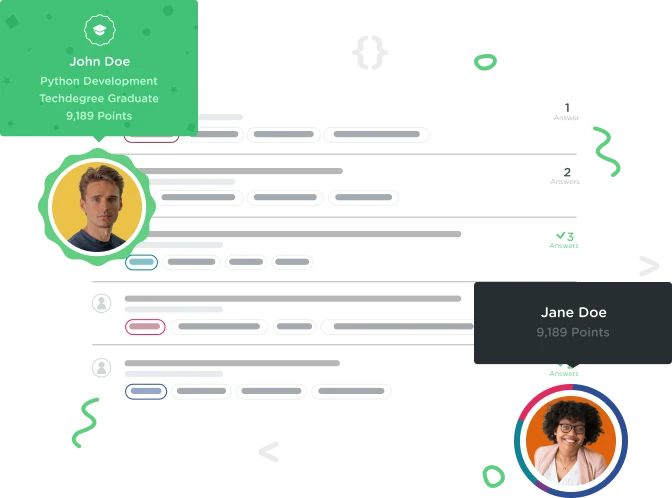

Larry Singleton
18,946 PointsUnit Testing in C#
Link: https://teamtreehouse.com/library/refactor
Question: Challenge Task 1 of 4
Stub out the Calculator class so that the code compiles, but the test cases in CalculatorTests fail as expected.
Calculator.cs is empty
CalculatorTests.cs
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
}
I've not added anything because in the videos there is NO reference on how to "Stub Out" anything. I have watched the videos several times and the word and definition is not mentioned. What is meant by "Stub Out"? Please feel free to go in behind me and find there reference if its there. I'm by no means perfect, if it's there, that's on me and I apologize... I'm not finding it and need some direction.
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
}
4 Answers
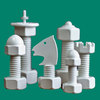
Steven Parker
231,275 PointsFor example, if I was asked to "stub out" a "swim" class that contained a method named "splash" that took a string argument and returned an integer, I might write this:
public class swim
{
public int splash(string arg)
{
return 0; // temp, needed to compile
}
}

Larry Singleton
18,946 PointsCan you please provide an example.

Larry Singleton
18,946 PointsThank you
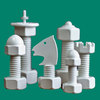
Steven Parker
231,275 PointsTo "stub out" is to create the structure but no contents.
So in this case you'll create the class itself and the methods inside it, but (for the moment) leave the methods empty so they don't do anything.
Any functional tests will fail, of course, but the challenge is expecting that for this task.