Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial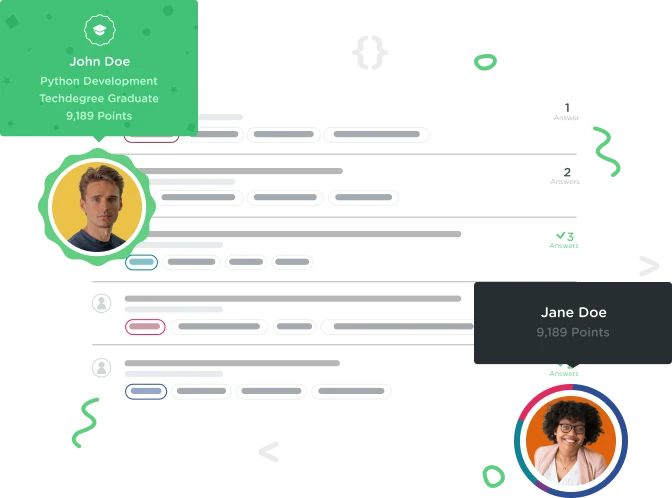
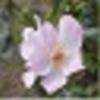
Katrin Nitsche
23,462 PointsUnit Testing in C# - Test Driven Development (Challenge Task 4 of 4)
I don't know how to pass this. I think is because the numbers are not equal like 0.900001 and 0.900002. I don't know how to fix. Could somebody give me a hand, please?
public class Calculator
{
public double Result;
public Calculator(double number)
{
Result = number;
}
public void Add(double number)
{
Result += number;
}
public void Substract(double number)
{
Result -= number;
}
}
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicSubtract()
{
var target = new Calculator(1.1);
target.Substract(0.2);
var expected = 0.9;
Assert.Equal(expected, target.Result);
}
}
1 Answer

James Churchill
Treehouse TeacherKatrin,
You're on the right track!
xUnit's Assert.Equal
has an overload with the following method signature:
void Assert.Equal(double expected, double actual, int precision)
This method "verifies that two double values are equal, within the number of decimal places given by precision".
Once you get past that issue, the code challenge runner will complain that task 3 isn't passing anymore. In order to resolve that issue I had to rename your Substract
method to Subtract
.
I hope this helps :)
Thanks ~James
Katrin Nitsche
23,462 PointsKatrin Nitsche
23,462 PointsHello James,
Thank you very much. It worked. Would a little typo can do to you. :-(
Katrin