Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial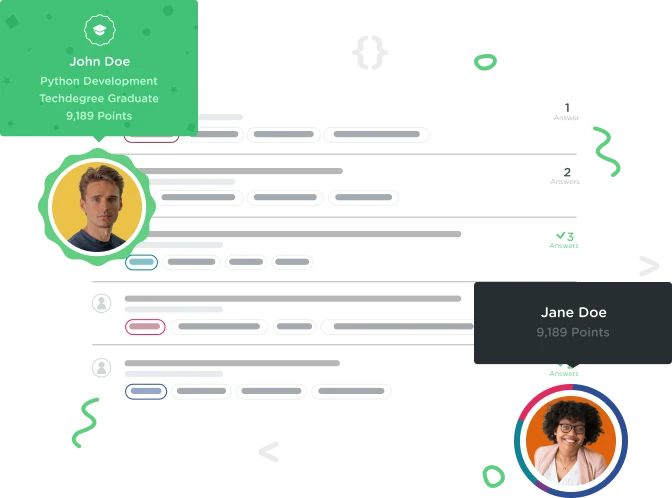
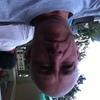
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 PointsUnittests : Hi there can someone look at the following set of tests.
The tests I have created work, however, I feel that I have missed the point as the tests could standalone without the need to import the actual project?
import datetime
import unittest
from peewee import *
import worklog
TEST_WORKLOGS = [
{
'Employee Name': 'Stuart McIntosh',
'Task Completed': 'Completed database',
'Date Started': '01/05/17 09:15',
'Date Completed': '10/05/17 11:23',
'Time Taken': 7680,
'Time String': '2.0 hours 8.0 minutes',
'Notes': 'Bunch of text'
},
{
'Employee Name': 'Milka McIntosh',
'Task Completed': 'Walked the dog',
'Date Started': '2017-05-01 12:15:00',
'Date Completed': '2017-05-01 12:45:00',
'Time Taken': 1800,
'Time String': '0.0 hours 30.0 minutes',
'Notes': 'Bunch of text',
}
]
class WorkLogTests(unittest.TestCase):
''''Main test class for WorkLog'''
def test_get_employee_name(self):
''' Tests to ensure we get a valid employee name back
from the user input, the first test check it matches a regex of
'firstname lastname'
'''
self.assertRegex(TEST_WORKLOGS[0]['Employee Name'], r'[\w]+\s[\w]+')
def test_get_task(self):
''' tests to ensure we get a valid task back from the user'''
self.assertGreaterEqual(len(TEST_WORKLOGS[0]['Task Completed']), 1)
self.assertLessEqual(len(TEST_WORKLOGS[0]['Task Completed']), 30)
def test_get_date_started(self):
''' test to ensure we have a valid date time from the user'''
date_started = datetime.datetime.strptime(TEST_WORKLOGS[0]
['Date Started'],
'%d/%m/%y %H:%M')
self.assertLessEqual(date_started,
datetime.datetime.now())
def test_get_date_completed(self):
''' test to ensure we have a valid date time from the user'''
date_completed = datetime.datetime.strptime(TEST_WORKLOGS[0]
['Date Completed'],
'%d/%m/%y %H:%M')
self.assertGreaterEqual(date_completed,
datetime.datetime.now())
if __name__ == '__main__':
unittest.main()
Added to the above what I think I need to do is to test user_input with Mock! Can someone point me to a simple explanation as to how that works.
Khem Myrick
Python Web Development Techdegree Graduate 18,701 PointsKhem Myrick
Python Web Development Techdegree Graduate 18,701 PointsI've also been looking for a simple explanation of Mock, especially in regards to the input() function and the peewee module. Anybody have a few pointers and/or links?