Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial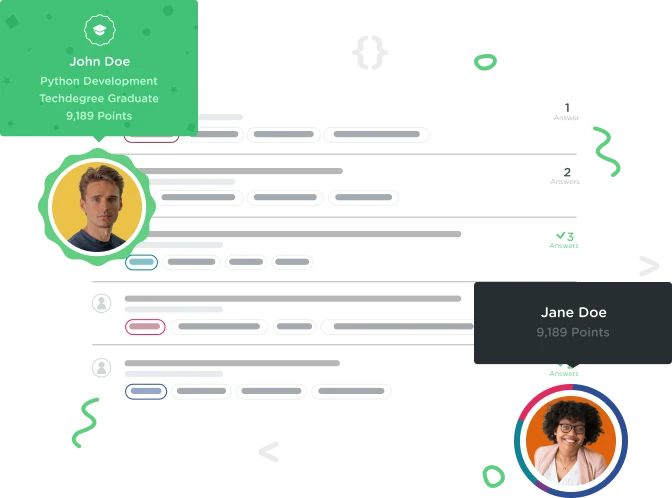

Evans Attafuah
18,277 PointsUnknown Error
Am not able to complete this challenge, something about an unknown error, is anyone experiencing this same problem?
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
public static final String TAG = MainActivity.class.getSimpleName();
public Button mButton1;
public Button mButton2;
/*
* Some code has been omitted for brevity
*/
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mButton1 = (Button)findViewById(R.id.button1);
mButton2 = (Button)findViewById(R.id.button2);
}
public void trackButtonPress() {
mButton1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this, "A button was pressed", Toast.LENGTH_LONG).show();
Log.i(TAG, "A button was pressed");
}
});
mButton2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this, "A button was pressed", Toast.LENGTH_LONG).show();
Log.i(TAG, "A button was pressed");
}
});
}
}
3 Answers

Ben Jakuben
Treehouse TeacherEvans, I'm sorry you're having such trouble with this one! I don't want to give the full answer here, but if this still doesn't help let me know and I'll send it to you privately.
Let's start over. This method definition just like you have it is a good start:
public void trackButtonPress() {
Clear out all the code you added and start with this blank method:
public void trackButtonPress() {
}
Next, all you need to paste in here are the two lines from either onClick() method. This is the point of this exercise: to turn those duplicate lines into a new method that you can then reuse.
public void trackButtonPress() {
// this will now have two lines of code that you copy/pasted
}
The last thing to do is replace both occurrences of those two lines with a call to this method instead. Instead of having those same two lines in two places, now you will have a simple method call in two places instead.
Hope this gets you past this challenge!
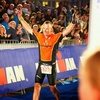
Steve Hunter
57,712 PointsHiya,
I think the onClickListeners need to stay there so that the click is picked up. However, the code inside the onClick
method is a repetition.
// this is inside onCreate()
mButton1 = (Button)findViewById(R.id.button1);
mButton2 = (Button)findViewById(R.id.button2);
// onClickListener ONE - in onCreate
mButton1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
trackButtonPress(); // this is the refactored function
}
});
// onClickListener TWO - in onCreate
mButton2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
trackButtonPress(); // this is the refactored function
}
});
} // <--- this is the end of onCreate()
// this is the refactored function - the onClickListeners stay in onCreate
public void trackButtonPress(){
// these are the only two duplicated lines, now refactored.
Toast.makeText(MainActivity.this, "A button was pressed", Toast.LENGTH_LONG).show();
Log.i(TAG, "A button was pressed");
}

Evans Attafuah
18,277 PointsDid you do the challenge relating to this post? it required that I just copy the code into trackButtonPress method. So I still don't know why it's not working. But thanks for your help.
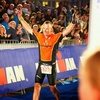
Steve Hunter
57,712 PointsHi,
Yes I did do the challenge so that I could understand the issue you were faced with. I did that then compared my answer with your code that you posted above.
It showed me that you had refactored too much code as you had pasted the two onClickListener
methods out of onCreate()
and into trackButtonPress()
. That would stop the code working.
The only two identical lines, using DRY principles, are the Toast
and the Log
lines.
If you compare your code to my suggested solution you will see that, whilst using the same lines of code, I have done it in a different order.
The solution isn't to just copy the code into trackButtonPress()
it is to copy the correct lines of code into there! You took too much with you, taking the onClick
out of where they need to be. Indeed, the trackButtonPress()
function would never be reached if the code that fires when a button is pressed is inside that method.
Hope that helps you get through the challenge.
Steve.
Evans Attafuah
18,277 PointsEvans Attafuah
18,277 PointsFinally got it. I was suppose to copy just the Toast and Log sections of the code into my trackButtonPress. The problem was I was trying to refactor the new View.OnClickListener method instead.