Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial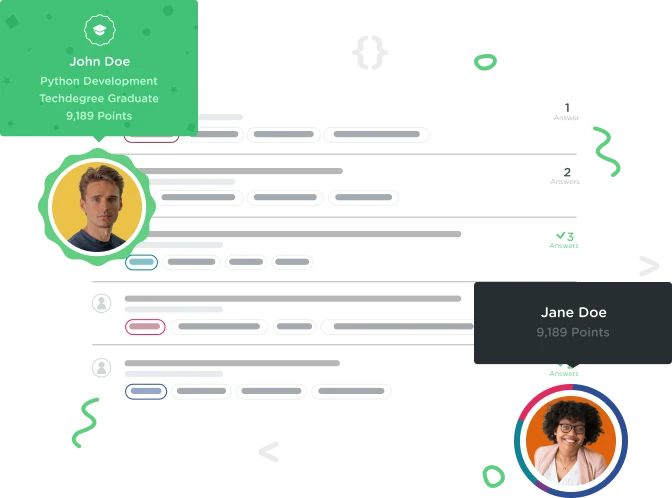

Noah Harness
1,383 PointsUnpacking
Let's test unpacking dictionaries in keyword arguments. You've used the string .format() method before to fill in blank placeholders. If you give the placeholder a name, though, like in template below, you fill it in through keyword arguments to .format(), like this: template.format(name="Kenneth", food="tacos") Write a function named string_factory that accepts a list of dictionaries as an argument. Return a new list of strings made by using ** for each dictionary in the list and the template string provided.
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"

Noah Harness
1,383 PointsOh no I have been trying for about an hour. I just deleted my work.....don't assume. I'm confused with the language.
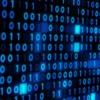
Alexander Davison
65,469 PointsWell... Can you try again and post your code so I can point out what you could do to fix it?
3 Answers
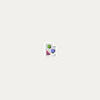
doesitmatter
12,885 PointsHey Noah,
I was facing the same problem, managed to figure it out though eventually, by having a look at the teachers note under unpacking. See solution below, if you absolutely can't figure it our.
Let me break down the challenge for you: you need to first write a function called string_factory. This function will receive a list of dictionaries. These dictionaries will look like this: {"name" : "some_name", "food" : "some_food"}
. You will need to loop through all of these and for each dictionary d
you need to first insert the data (of name and food) into the template
string using format(**d)
then finally you add this to a list and when you've went through all the dictionaries, you return the list.
The trickiest part is actually this: template.format(**d)
, what **
does is unpack all the variables (food
and name
) and place this inside of the template string which has a placeholder for each value: "Hi, I'm {name} and I love to eat {food}!"
.
The complete code:
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dict):
new_list = []
for d in list_of_dict:
new_list.append(template.format(**d))
return new_list
Note that another instance in which the same notation: **
is used (which is confusing kind of) for packing. In packing the opposite concept applies, you are provided with key value pairs which you can all put into a dictionary. See the example below:
def packme(**kvs):
return kvs;
print(packme(first_name="John", last_name="Smith"))
#this will print: {'first_name': 'John', 'last_name': 'Smith'}
It is recommended to write key-value pairs without any space in between i.e. first_name="John"
, not first_name = "John"
.

Akbar Tahir
Courses Plus Student 2,060 Pointsits correct
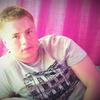
Mischa Yartsev
20,562 PointsHey Noah Harness
Here you should use a for loop to iterate over a dictionary, like so:
# initiate an empty list, to hold a list of dictionaries
my_list = []
for value in list_dict:
my_list.append(#put here a template placeholder)
where 'list_dict' should be a List of Dictionaries or an argument of the function. also, don't forget to put the code in function as it was required in a task.
Let me know if you need some additional help. Cheers!
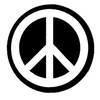
john larson
16,594 PointsHey Mischa, that was nicely done without giving it all away.
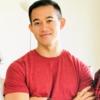
Xayaseth Boudsady
21,951 PointsHere's a simpler way based on the video.
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(**dict)
Just use the ** (asterisk) to unpack your dictionary into those template.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsPlease try your best before reaching out to the Community. If you never try, you will never succeed.
I would also re-watch the videos that I didn't understand completely at first try.