Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial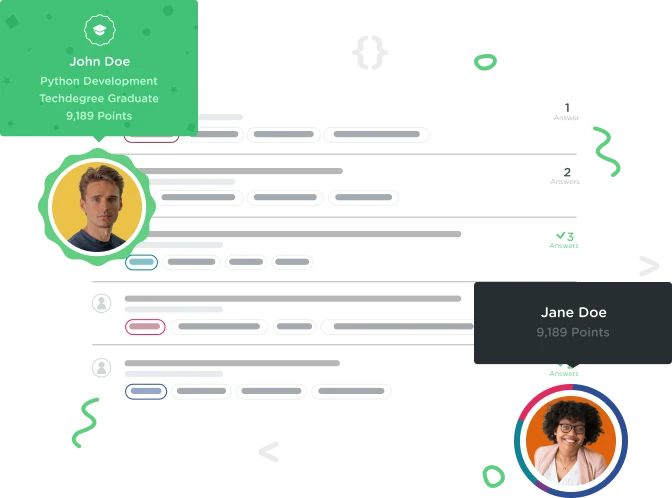

sowmya c
6,966 Pointsunpacking a dictionary
we have to return a new string using the string_factory and template
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
def string_factory(**kwargs):
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
template = "Hi, I'm {name} and I love to eat {food}!"
new_list = []
new_list.append (template.format(values["name"], values["food"]))
return new_list
3 Answers
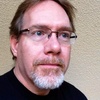
Chris Freeman
Treehouse Moderator 68,423 PointsYou have many of the correct ideas.
Remember that the function takes a single argument that will be similar to the values
list shown in the challenge code comments. Don't overwrite the values argument by redefining it the inside of the function. Your function should:
- loop through the
values
list - use each dict to format the
template
string - return a list of these formatted strings
Post back if you need more help. Good luck!!!

Elad Ohana
24,456 PointsHi,
You do not need to add a values variable for the challenge, it is designed to be passed into your function. Since you are looking for a dictionary to be passed in, you should not put the ** as part of the **kwargs argument, since the function will try to interpret what's in the values themselves, instead of using them as the list of the dictionaries. Also, the names are defined in the template string as well as in the dictionary that will be passed into kwargs. Finally, you are looking to return a list of multiple strings with the values filled in, which can't be done with a single append statement.

sowmya c
6,966 Pointsdef string_factory():
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
template = "Hi, I'm {name} and I love to eat {food}!"
sow = []
for value in values:
sow = template.format(**name, **food)
return sow
THIS IS ALSO GIVING ERROR
[MOD: added ```python formatting -cf]
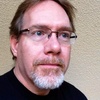
Chris Freeman
Treehouse Moderator 68,423 PointsYou're almost there. Remember to keep the parameter values
in the definition and not redefine it inside the function. Also
- When using format, use the argument
**value
- use
.append
when assigningsow
so that a list is returned