Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial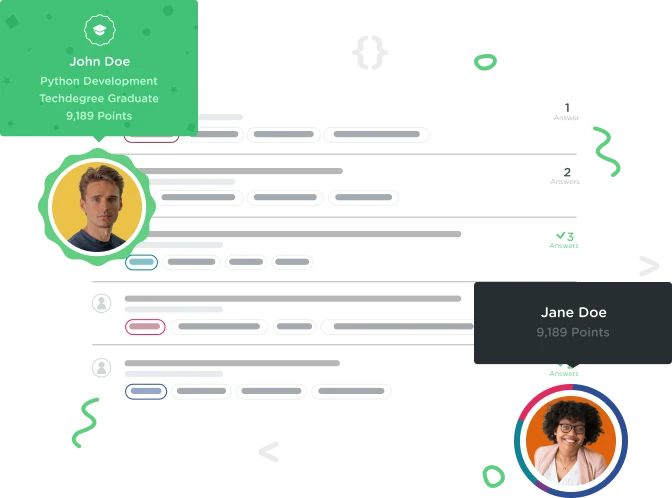

Shazia Ali
Python Development Techdegree Student 1,436 PointsUnpacking dictionaries
Can someone explain unpacking dictionaries in some details. I don't understand how I can get both keys and values. Calling the function with print_teacher(**teacher) only returns values. How can i get keys as well
5 Answers
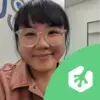
Rachel Johnson
Treehouse TeacherTo answer your question directly, you can get the values and keys by using teacher.items()
.
However, in the context of this step, unpacking teachers with **teachers
and feeding it directly into the function means you won't see the keys or values unless you print them out.
**teachers
is essentially feeding the following into the function:
name="Ashley", job="Instructor", topic="Python"
This matches the 3 keyword arguments of print_teacher()
.
Then, the 3 values are printed out because of the 3 print statements. We don't see the keys because they're being used to tell the function which values are matched with what keyword argument

jhon white
20,006 Pointsteacher = {
'name':'Ashley',
'job':'Instructor',
'topic':'Python'
}
def print_teacher(name, job, topic):
print(name)
print(job)
print(topic)
# when you pass the keyword (**"dic name")to a function, it unpack the dic to (key, value )
#like ('name':'Ashley') and assign it to the function parameter.
print_teacher(**teacher)
Good luck :)

jhon white
20,006 PointsBecause of the values been assigned to your function parameters.
if you wanna get the key and the value pair, you can use this method:
teacher = {
'name':'Ashley',
'job':'Instructor',
'topic':'Python'
}
def print_teacher(dict):
for key in dict:
print("key:", key, ", Value:", dict[key])
print_teacher(teacher)
Good luck :)

Parker Higgins
6,277 PointsWhen you call print_teacher(**teacher), the **teacher is unpacking the dictionary, and turning it's contents into keyword argument:value pairs. It creates a keyword argument for each pair, with the name of the argument being the key, and the value being the value. Since you created def print_teacher(name, job, topic): you already know what all the keyword names are, they are name, job, topic.

Shazia Ali
Python Development Techdegree Student 1,436 PointsThanks for your reply. When I called the function - print_teacher(**teacher) - I didn't return a key, value pair. it only returned the values? How will I get the keys?