Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial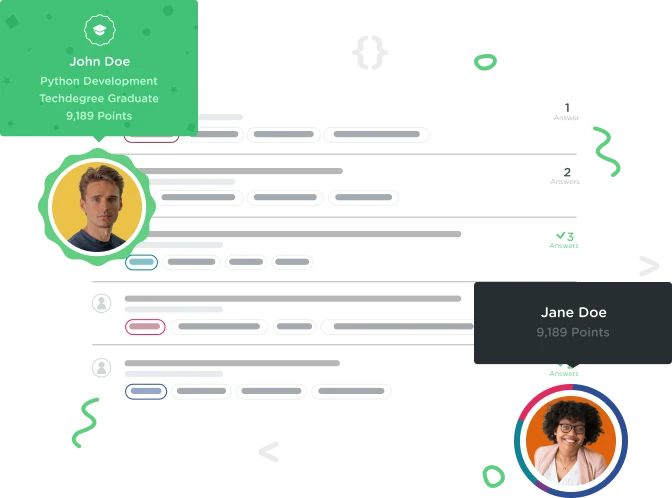

linda wu
3,016 PointsUnpacking dictionary
dict = {'name': 'Michelangelo', 'food' : 'PIZZA'} def favorite_food(name = 0, food = 0): if (name and food): result = "Hi, I'm {} and I love to eat {}!".format(name, food) return result
print favorite_food(**dict)
dict = {'name': 'Michelangelo', 'food' : 'PIZZA'}
def favorite_food(name = 0, food = 0):
if (name and food):
result = "Hi, I'm {} and I love to eat {}!".format(name, food)
return result
favorite_food(**dict)
5 Answers
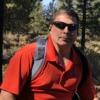
Mark Kastelic
8,147 PointsHi Linda,
Your solution works as written, but the challenge states that the function must accept a dictionary. Your function currently has two keyword arguments. When you call it by passing **dict, you are unpacking dict to fill in the two keyword arguments. That works. But, to set up the function to actually accept a dictionary object directly, you have to do something like this:
def favorite_food(dict):
values = []
for item in dict.items():
values.append(item[1])
return "Hi, I'm {name} and I love to eat {food}!".format(name=values[0], food=values[1])

linda wu
3,016 PointsCool, thanks Mark for your explanation! I got it now.
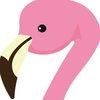
Dave StSomeWhere
19,870 PointsI'm pretty sure that the goal of the challenge is to understand the spiffy feature unpacking a dictionary while passing it to the format()
function call.
So while the code above works, the unpacking feature makes it much simpler - no need to loop and append into a list.
Here's the code using unpacking
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(**dict)

linda wu
3,016 PointsThis is really neat Dave! I love it.
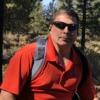
Mark Kastelic
8,147 PointsYou are right, unpacking should be retained in the challenge. Thanks for that beautiful Pythonic refinement! I will now go back to my REPL and "import this" ten times :).

linda wu
3,016 PointsI do spent good amount of time to complete this challenge. I think the instructor's intension was understand both dictionary unpacking as well as string.format function with python. However, the video was not very tailor made to tackle this challenge and it seems made a lot of people stumble on this question.
I found this link is quite helpful. https://www.python-course.eu/python3_formatted_output.php
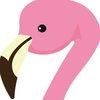
Dave StSomeWhere
19,870 PointsNice link thanks, those place holders are always confusing to me, so I rarely use them and have to look them up all the time -
linda wu
3,016 Pointslinda wu
3,016 PointsHi,
I don't understand why this is failing for me. I ran the code in Pycharm and it works totally fine. However, it is always failing in treehouse. Someone please help me to understand why it is not working. Thanks