Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial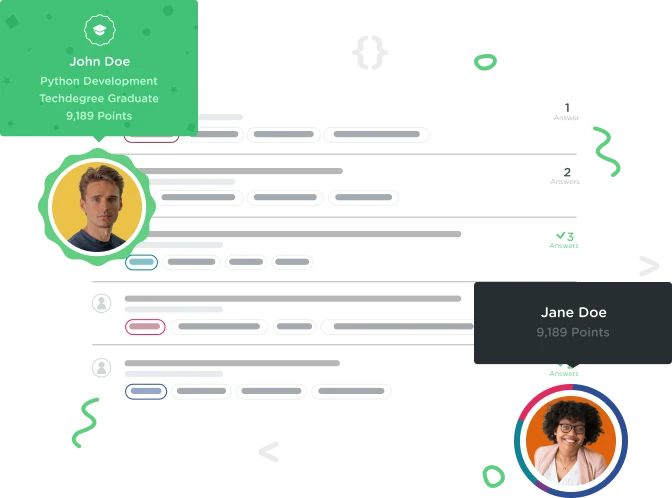

mk Eagle
18,595 PointsUnreported exception
I'm trying to run this test in IntelliJ just as Craig runs his, but mine keeps producing this error:
Error:(22, 69) java: unreported exception com.teamtreehouse.vending.InvalidLocationException; must be caught or declared to be thrown
Any thoughts on what I'm doing wrong?
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* Created by mk.eagle on 11/2/2016.
*/
public class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
@Before
public void setUp() throws Exception {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
public void validInputReturnsProperLocation() throws Exception {
AlphaNumericChooser.Location loc = chooser.locationFromInput("B4");
assertEquals(1, loc.getRow());
assertEquals(3, loc.getColumn());
}
}
3 Answers

mk Eagle
18,595 PointsHALLELUJAH. After much poring over code, I discovered that my problem was in InvalidLocationException. Although I don't remember ever changing this, I had "Throwable" instead of "Exception" :
package com.teamtreehouse.vending;
public class InvalidLocationException extends Exception {
public InvalidLocationException(String s) {
super(s);
}
}
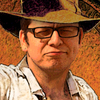
Jose Salazar
9,393 PointsThank you!

mk Eagle
18,595 PointsI didn't change anything in AlphaNumericChooser. This is my code:
package com.teamtreehouse.vending;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class AlphaNumericChooser extends AbstractChooser {
private int ALPHA_FIRST = (int) 'A';
private int ALPHA_LAST = (int) 'Z';
public AlphaNumericChooser(int maxRows, int maxColumns) {
super(maxRows, maxColumns);
int maxAllowedAlpha = (ALPHA_LAST - ALPHA_FIRST) + 1;
if (maxRows > maxAllowedAlpha) {
throw new IllegalArgumentException("Maximum rows supported is " + maxAllowedAlpha);
}
}
@Override
public Location locationFromInput(String input) throws InvalidLocationException {
Pattern pattern = Pattern.compile("^(?<row>[a-zA-Z]{1})(?<column>[0-9]+)$");
Matcher matcher = pattern.matcher(input);
if (!matcher.matches()) {
throw new InvalidLocationException("Invalid buttons");
}
int row = inputAsRow(matcher.group("row"));
int column = inputAsColumn(matcher.group("column"));
return new Location(row, column);
}
private int inputAsRow(String rowInput) {
rowInput = rowInput.toUpperCase();
return (int) rowInput.charAt(0) - ALPHA_FIRST;
}
private int inputAsColumn(String columnInput) {
int columnAsInt = Integer.parseInt(columnInput);
return columnAsInt - 1;
}
}

mk Eagle
18,595 Points(I should add that I had some trouble updating to the latest IntelliJ version, so instead I installed the same version Craig's using in the videos. I had difficulty with GitHub so I opened the project files from the downloads instead.)
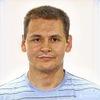
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 Pointsshow me the screenshot with error, so that I can see simultaneously test class and console output

mk Eagle
18,595 Points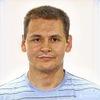
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsNot enough, show me Test class itself.
Like I said, it is a compiler error, so I need to see what intellijidea says about your code.
There should be red squiggly line 22
You should solve this error by adding throws Exception

mk Eagle
18,595 PointsOh, whoops. I see what you mean now. Here's the full screen.

Simon Coates
28,694 PointsI finally got around to doing this course. The Exception not extending Exception was always going to cause people trouble (unsure why Craig Dennis would write it that way). I just altered the test method signature. seems to work okay for now.
@Test
public void validInputReturnsProperLocation() throws Exception, Throwable {//mod
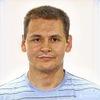
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsWhy do you ever want it to be Throwable
when in Craigs InvalidLocationException class repo for this course
is Exception
...? How did you get it to be Throwable
in the first place. There is no need for that ...

Simon Coates
28,694 PointsHi Alexander Nikiforov , some of the download code for the video has:
package com.teamtreehouse.vending;
public class InvalidLocationException extends Throwable {
public InvalidLocationException(String s) {
super(s);
}
}
I think he switches over to use exception, but as I started following from the s2v2 code that Craig Dennis posted (under downloads), the code I had used throwable.
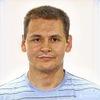
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsOk. Makes sense then.
I still think the better way is to change Throwable
to Exception
, because the way you write two Exceptions in the @Test
is not the best practice.
public void validInputReturnsProperLocation() throws Exception, Throwable {//mod
}
Read here for best practices, that says that we better leave one throws
not many.
And here is the StackOverflow post about why we better avoid Throwable
Quoting
Always throw an Exception (never a Throwable). You generally don't catch Throwable either, but you can. Throwable is the superclass to Exception and Error, so you would catch Throwable if you wanted to not only catch Exceptions but Errors, that's the point in having it. The thing is, Errors are generally things which a normal application wouldn't and shouldn't catch, so just use Exception unless you have a specific reason to use Throwable.

Simon Coates
28,694 PointsHi Alexander Nikiforov, thanks for the additional information. good to know. It did occur to me later that I could just use 'throws Throwable' and omit the redundant subclass.
Simon Coates
28,694 PointsSimon Coates
28,694 PointsNot an expert, but it sounds like you have a throw statement, without this being immediately caught or featuring in a method with a throws in the definition. can you post the AlphaNumericChooser code?