Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial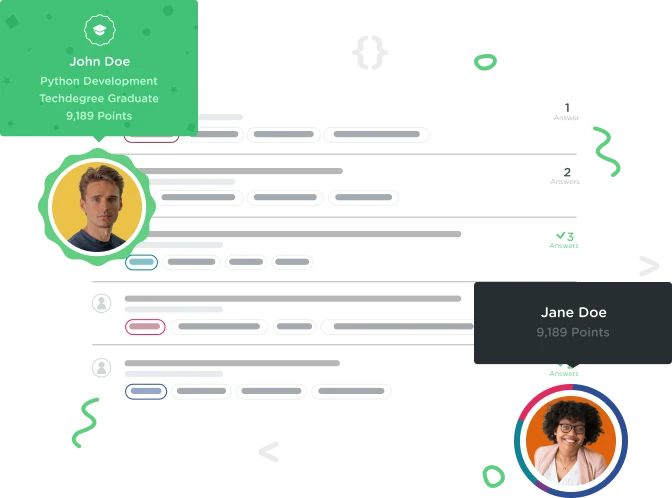

micheleg
3,808 PointsUnsure about this Arrays task
My brain just isn't registering this, I can't figure out how to do it. I've been following along but now I'm lost. Any explanations much appreciated. This is the question:
Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console.
Thanks
var temperatures = [100,90,99,80,70,65,30,10];
for ( i = 0; i >= 10; i += 1 ) {
console.log( temperatures );
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Leandro Botella Penalva
17,618 PointsHi micheleg,
In the array there are 8 items in total and you want to loop through that items. First you need to know that arrays start to count from 0 so the first item corresponds to the index 0 and the last items to the index 7. The the loop would be "loop starting from index 0 while the index is less or equal to 7 (last index)" and that in code would be:
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i <= 7; i++) {
console.log(temperatures[i]);
}
To read a value from an array you use the array name and between square brackets you put the index or position you want to get, remember first position is always 0.
In the task you know how many items has the array but imagine you don't know the size of the array. Another way would be
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}
.length returns the number of items of an array, in this case you use less than but not equals to because the size is always one unit above the last index. In an array of 8 items, .length would return 8 but the last item index is 7 so we don't want to include the index 8 since it doesn't exist.
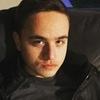
Emre Karakuz
9,162 PointsFirst of all, the variable 'i' inside for loop should have proper limit for not indexing the elements outside of the loop. If an array have 10 items, varible 'i' should change 0 to 9. not 10 or something else.
In your code, 'i' starts from zero but the loop will run when i >= 10. Because of having a zero value in 'i', loop will never run.
To adjust proper limit for 'for' loop, use .length. its always better.
for ( i = 0; i < temperatures.length ; i += 1 )
in addition, you are targeting all of the array 'temperatures' with the console.log To target each item in the array, add [i] after temperatures like this :
console.log( temperatures[i] );

micheleg
3,808 PointsThank you! This is starting to make sense. :)
micheleg
3,808 Pointsmicheleg
3,808 PointsThank you so much! This makes sense and is actually simpler than I expected. Thank you for taking all this time.