Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial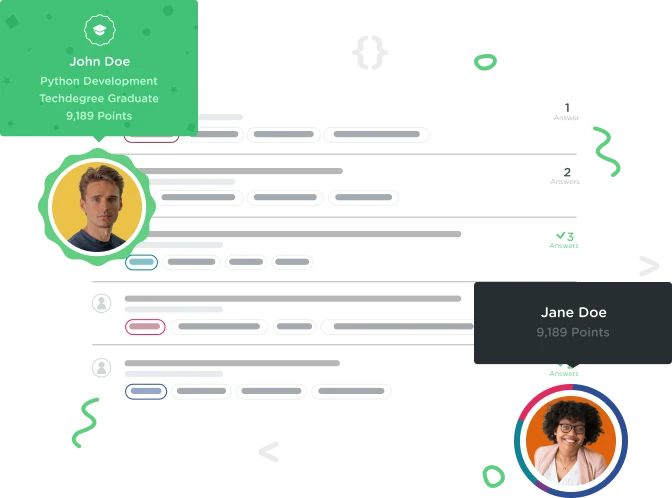

Michael Bau
3,394 PointsUnsure how to use 'Double' values
Hi,
I can't get this to work. I am not sure of the syntax in the switch statement when trying switch on 'double' values'. How do you actually write it out? I have tried (among many others) with:
case "Eiffel Tower": (48.8582, 2.2945) (as an example), but that doesn't work
What is the correct syntax when dealing with doubles?
Furthermore, how do you return the values when you are not supposed to label the return arguments?
Best regards, Michael
// Enter your code below
func coordinates(for location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": (48.8582, 2.2945)
case "Great Pyramid": (29.9792, 31.1344)
case "Sydney Opera House": (33.8587, 151.2140)
default: (0.0)
}
return (location)
}
let locationResult = coordinates(for: "Eiffel Tower")
2 Answers
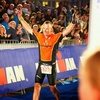
Steve Hunter
57,712 PointsHi Michael,
You're pretty much right with that but you want to return
your tuples, not location
as that is what gets passed into the func.
So, just add a return
in front of the tuples and remove your existing return
line:
switch location{
case "Eiffel Tower": return (48.8582, 2.2945)
case "Great Pyramid": return (29.9792, 31.1344)
case "Sydney Opera House": return (33.8587, 151.2140)
default: return (0, 0)
}
So, the user passes in "Sydney Opera House" as the string, and the method returns the tuple, (Double, Double)
.
Make sense?
Steve.
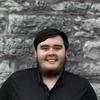
Michael Hulet
47,913 PointsThere's nothing special about switch
ing on Double
values in Swift. In fact, your handling of all the Double
s looks just fine. The reason you're failing right now is because you're not actually doing anything with any of the values in the switch
statement, but the challenge asks you to return
them. Currently, your code doesn't do anything with any of the tuples it creates, and then return
s the location
parameter without modifying it. Instead, you need to write return
before every tuple in the switch
statement, and not at the bottom of the function. Lastly, you'll need to specify 0
twice in the default
case, for both the latitude and longitude. You've left all the values in all your tuples unnamed so far like the challenge asks, so once you fix the problems mentioned above, you should pass the challenge. Great job!

Michael Bau
3,394 PointsHi Michael,
Thanks a lot for your help. I guess I was too focused on the 'double' values there and forgot all about actually returning the values.
Best regards, Michael
Michael Bau
3,394 PointsMichael Bau
3,394 PointsHi Steve,
Once again, thanks for your help! I wasn't aware that I were to return a value for each case, but now I know :o)
Best regards, Michael
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Michael,
No problem - glad it made sense and you got through it.
Returning an unnamed thing can feel odd at times; we so often return a named constant or variable - and tuples are often an unfamiliar construct too, which can add to the confusion.
Giving it some thought, I wondered whether assigning the tuples to a variable/constant (a named thing) then returning that - would this add clarity, make the code more readable or just cut through some of the confusion? I don't think it did:
I could probably (certainly!) have done a better job of naming that constant but I don't feel that this code is any clearer. The addition hasn't cut through the confusing areas, indeed, it has probably diverted attention away from where it is needed! It was worth a try, though. But, as is a common theme with my code, it failed to deliver anything better - these are just concepts that need familiarity to get through the confusion. Dressing the code up doesn't seem to, I don't think.
Your thoughts?
Steve.