Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial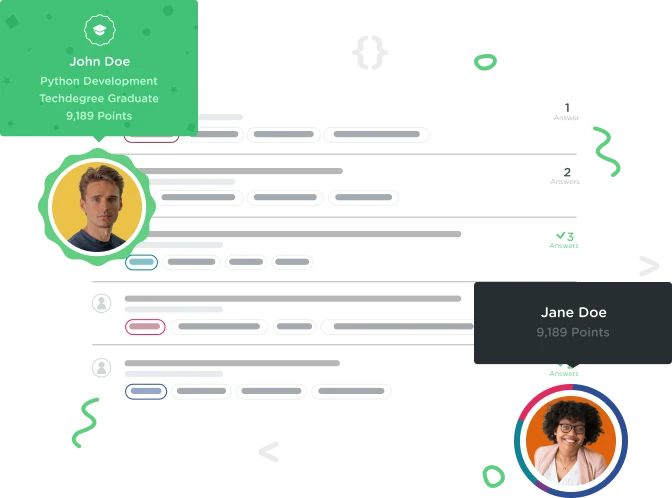
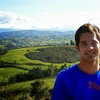
Dhruv Ghulati
1,582 PointsUnsure of how to create a tuple of pairs
I am trying to loop through 2 iterables, and go through the indices of each iterable, and pair up the values for those indices. Those pairs created will then be appended to an empty tuple, and that tuple will be appended to an empty list. However, here I get "list object doesn't have an enumerate attribute". What am I missing here? Thanks
# combo(['swallow', 'snake', 'parrot'], 'abc')
# Output:
# [('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
# If you use list.append(), you'll want to pass it a tuple of new values.
# Using enumerate() here can save you a variable or two.
def combo(iter1,iter2):
output=list()
for index,value in iter1.enumerate(),iter2.enumerate():
pairs=tuple()
pairs.append(iter1(value),iter2(value))
output.append(pairs)
return output
2 Answers
William Li
Courses Plus Student 26,868 PointsThere're some issues with your solutions.
-
for index,value in iter1.enumerate(),iter2.enumerate():
, I can see that you're trying to iterate through TWO iterables in parallel, but it's illegal to write it like that and it is incorrect way of using enumerate build-in function, you can only write itfor index,value in enumerate(iter1):
, this will loop through iter1 and return each of its item along with index. -
pairs.append(iter1(value),iter2(value))
, you can not append item to a tuple, as tuple is immutable.
Actually this problem tells you that
Assume the iterables will be the same length.
This assumption should make writing the function quite a bit easier, because you now only need to have one loop to loop through both iterables using index value 1, 2, ,3 ...
.
def combo(iter1,iter2):
output=[]
for index in range(len(iter1)): # 1, 2, 3 ... len(iter1)-1
# group (iter1[index],iter2[index]) in a tuple and append it to output list
output.append( (iter1[index],iter2[index]) )
return output
OR, make use of enumerate()
.
def combo(iter1,iter2):
output=[]
for index, value in enumerate(iter1):
output.append( (value, iter2[index]) )
return output
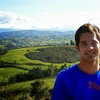
Dhruv Ghulati
1,582 PointsThank you for this. For some reason on stack exchange it said you can append items to a tuple, but yes I get the immutability aspect point.
I think your second solution where you effectively append a pair each time is helpful!
Thanks
William Li
Courses Plus Student 26,868 PointsYep, use whichever approach you like better. tuple in Python is immutable data structure, but if you really wanna make change to a tuple, you can convert it to a list by using list()
method.