Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial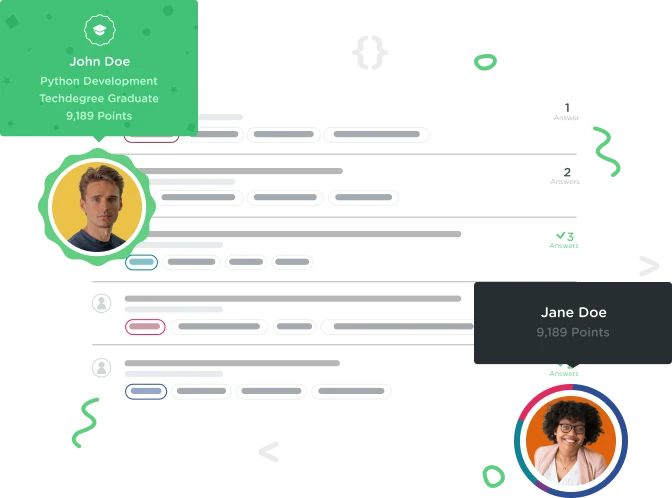
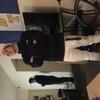
Brandon Hoffman
3,642 PointsUnsure of what I am comparing in the doubles function (hands.py)
So the double property is supposed to compare two rolls and see if they get the same value and return true if so, but I am confused what I declare to get the values/instances of those two rolls within the double property. Can someone help point me in the right direction?
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size = 2, die_class = D6)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
@property
def doubles(self):
@classmethod
def reroll(self):
ch = CapitalismHand()
return ch
1 Answer
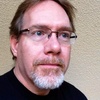
Chris Freeman
Treehouse Moderator 68,441 Pointsself
is the list of current die values. Doubles would be if the first two items in the list were equal. The notation looks strange, but you can reference the die using self[0]
and self[1]
.
Post back if you need more help. Good luck!!!
Clifford Gagliardo
Courses Plus Student 15,069 PointsClifford Gagliardo
Courses Plus Student 15,069 PointsCould you explain this further? I don't understand the principle behind that solution.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsTravel down the rabbit hole as deep as you wish....
The class
CapitalismHand
inherits fromHand
which inherits fromList
so it's behavior is an extension of a regular list. Since thesize
attribute lead to creating a list of that length, doubles can be determined by looking at the first two elements in the list itself. Theself
variable is a pointer to this instance ofCapitalismHand
which is the list.All attributes and methods of
list
are available toCapitalismHand
, as is slicing and index notation. Soself[0]
andself[1]
are the first two elements of the list. These elements are instances of theD6
class. This means that any attributes such asself[0].value
refer to the D6 instance value, and any conversion calls such asint(self[0])
will run theD6.__int__
method.Now to the comparison. The flow below may seem a bit convoluted.
A class must have (or inherit) an
__eq__
method for comparisons to work. As seen the earlier Comparing and Combining Die video, The classD6
inherits fromDie
which gets both an__eq__
and an__int__
method added around 01:18 in the video.Semantics notes:
self
is theCapitalismHand
instance,self[0]
andself[1]
is are first two elements of the list where each is an instance of theD6
class, andother
is whatever object is being compared to.With these methods, two
D6
instances can be compared, such as,self[0] == self[1]
as follows:self[0].__eq__
will be called withself[1]
as other.int(self) == other
self[0].__int__()
on "self" (the first D6 instance) which returns the integerself.value
(the first D6 instance value)self.value
is an int 5, which leaves5 == other
("other" in this case is the second D6 instance)self[1].__eq__
to be called with the second die as "self" and the "5" as other.int(self) == other
self[1].__int__()
on "self" (the second D6 instance) which returns the integerself.value
(the second D6 instance value)self.value
is an int 4, which now leaves4 == 5
, which fails, ultimately returningFalse