Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial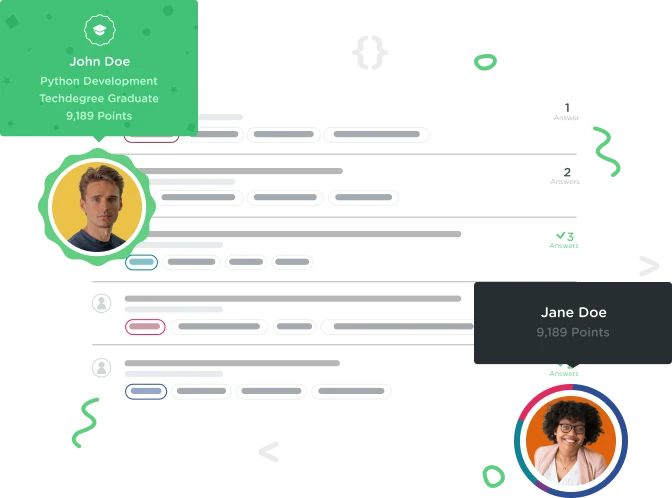

Tony Kirkland
2,400 PointsUnsure why I am getting 'try again'
In Python Collections I have the following task:
Create a function named nchoices() that takes an iterable and an integer. The function should return a list of n random items from the iterable where n is the integer. Duplicates are allowed.
My code:
def nchoices(choice_count, my_iter):
random_list = []
while choice_count > 0:
random_list.append(random.choice(my_iter))
choice_count -= 1
return random_list
My test (using Pycharm) was to call nchoices as follows:
grid = list(range(30)) print(nchoices(5, grid))
Results appears to be within the expected set:
[6, 24, 0, 7, 20] [20, 26, 1, 19, 25] etc.
Am I misreading the question? I get no errors with executing the code but it doesn't pass the check work validation.
Thanks in advance for your help!
4 Answers
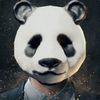
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi Tony, there are just a couple of things wrong with your code. Firstly, the order of the arguments passed in should be the iterable then the number. Secondly, you forgot to import random. Also, the challenge asks you to pick something out of the iterable for each number in range(the_number_you_passed_in)
. This can be done with the following code:
import random
def nchoices(my_iter, choice_count): #you should have passed arguments in this way round
random_list = []
for i in range(choice_count):
random_list.append(random.choice(my_iter))
return random_list
If you have any further questions, please feel free to leave a comment. :)
Thanks,
Haider
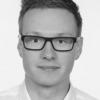
Urs Merkel
Courses Plus Student 4,552 PointsHi guys, could someone please explain me, why you are using 'while' and decrease it within your for loop. Shouldn't the for loop already do its job?
Haider Ali – you are decreasing the variable in your for loop, you use as an for-loop-argument, why is that so? Shouldn't it be working with just the for-loop?
Thanks for your help
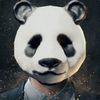
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi there Urs, thank you for pointing that out. The answer to your question is yes, the loop will run just fine without the decreasing the counter. Now that I look at my code, I have no idea why I have put that there, I must have thought I was using a while loop for some reason. Thats what I get for rushing my answers! :)
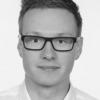
Urs Merkel
Courses Plus Student 4,552 PointsThanks Haider for your quick reply and pointing it out, I was just confused :D and unsure what the deeper meaning of this could be.
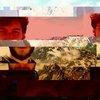
Gianmarco Mazzoran
22,076 PointsHi,
I think it's because you need a for loop in order to randomly appends items to the random_list
.
Here's my code (that's ok for the challenge):
import random
def nchoices(iterable, integer):
rand_list = []
for item in iterable:
while integer > 0:
rand_list.append(random.choice(item))
integer -= 1
return rand_list
Tony Kirkland
2,400 PointsTony Kirkland
2,400 PointsIs the reasoning behind the ordering of the parameters to the function as simple as how it was worded in the challenge? Functionally the order passed shouldn't matter as long as the function uses the values passed correctly. Correct?
As for the import I got that a bit later.. Seem a bit ambiguous in wording though as the challenge just says 'create the function' and normally one wouldn't import within the function creation. Explains why it was working in Pycharm though as I was importing there.
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHaider Ali
Python Development Techdegree Graduate 24,728 PointsHi Tony, I understand where you are coming from, I will show you how the order of passing in parameters can affect your code. For example, if I had the following function:
This would print the string out as many times as
a_number
. E.g. if i called the following code, this is what would happen:However, if I ran this:
example(3, 'hello')
I would get a
TypeError
saying that an str object cannot be interpreted as an integer. This is because functions use parameters in the order you pass them in so it would use3
as the string and'hello'
as the number. This can be a little confusing at first but having a little play around in the python shell should clear things up for you :).Tony Kirkland
2,400 PointsTony Kirkland
2,400 PointsI understand. As long as the order that the parameters are passed in the function call match the order that the that the function is expecting them in then it is correct. One must be sure that the orders match tho or as you stated there will be errors (or worse unexpected results) in the code.