Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial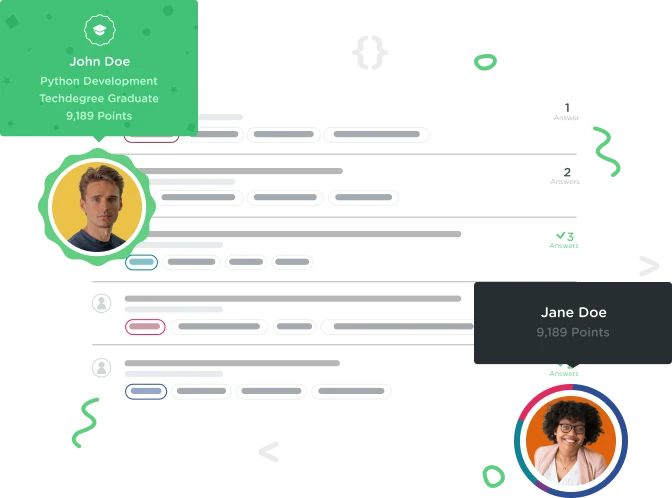

Mihai-Ady Zvancu
7,657 Points"Up" and "Down" buttons aren't working for the new list items while "Remove" buttons work for all list items.
For the sake of learning I've added those 3 buttons for the new items as well. But they don't work for the new items except for the "remove" buttons. That's strange. What am I doing wrong? Here is my JS code followed by my HTML. UPDATE: I saw in the next video what I'm currently doing but in another way. I still want to know what I am doing wrong. Thank you!
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
const show_or_hide = document.getElementById("show_or_hide");
const div = document.getElementById("div");
let new_title = document.querySelector("input");
const change_title = document.getElementById("change_title");
const p = document.getElementById("list_title");
let new_item = document.getElementById("new_item");
const insert = document.getElementById("insert");
const listUL = document.querySelector("ul");
show_or_hide.addEventListener("click", () => {
if(div.style.display === 'block'){
show_or_hide.textContent = "Show list";
div.style.display = "none";
}else{
show_or_hide.textContent = "Hide list";
div.style.display = "block";
}
});
change_title.addEventListener("click",() => {
p.textContent = new_title.value+":";
});
insert.addEventListener('click', () => {
let li = document.createElement('li');
li.textContent = new_item.value;
let up_button = document.createElement("button");
up_button.textContent = "Up";
up_button.className = "up";
li.appendChild(up_button);
let down_button = document.createElement("button");
down_button.textContent = "Down";
up_button.className = "down";
li.appendChild(down_button);
let remove_button = document.createElement("button");
remove_button.textContent = "Remove";
remove_button.className="remove";
li.appendChild(remove_button);
listUL.appendChild(li);
new_item.value = ""; });
listUL.addEventListener("click", (event) => {
if(event.target.className == "remove"){
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
});
listUL.addEventListener("click", (event) => {
if(event.target.className == "up"){
let li = event.target.parentNode;
let prev_li = li.previousElementSibling;
let ul = li.parentNode;
if(prev_li){
ul.insertBefore(li,prev_li);
}
}
});
listUL.addEventListener("click", (event) => {
if(event.target.className == "down"){
let li = event.target.parentNode;
let next_li = li.nextElementSibling;
let ul = li.parentNode;
if(next_li){
ul.insertBefore(next_li,li);
}
}
});
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
<!DOCTYPE html> <html> <head> <title>JavaScript and the DOM</title> <link rel="stylesheet" href="css/style.css"> </head> <body> <h1 id="myHeading">JavaScript and the DOM</h1> <p>Making a web page interactive</p>
<button id='show_or_hide'>Hide list</button>
<div id="div">
<input type='text'>
<button id="change_title">Change List Title</button>
<p id="list_title">Things that are purple:</p>
<ul>
<li>grapes
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Remove</button>
</li>
<li>amethyst
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Remove</button>
</li>
<li>lavender
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Remove</button>
</li>
<li>plums
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Remove</button>
</li>
</ul>
<input type="text" id="new_item">
<button id="insert">Insert item</button>
</div>
<script src="app.js"></script>
</body> </html>
1 Answer
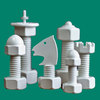
Steven Parker
231,275 PointsWhen posting code to the forum, use Markdown formatting to preserve the appearance, or share the entire workspace by making a snapshot and posting the link to it.
The button operation is triggered by element class, and the "down" button is never assigned a class. The "up" button is initially assigned the correct class, but a few lines later it is overwritten:
up_button.className = "down";
So the "up" button does work, but to move the line down.
Mihai-Ady Zvancu
7,657 PointsMihai-Ady Zvancu
7,657 PointsThank you a lot, sir! I need to improve my attention. I knew it will be annoying for reading but I didn't know how to format the text. Now I know. Thank you once again for your help.