Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial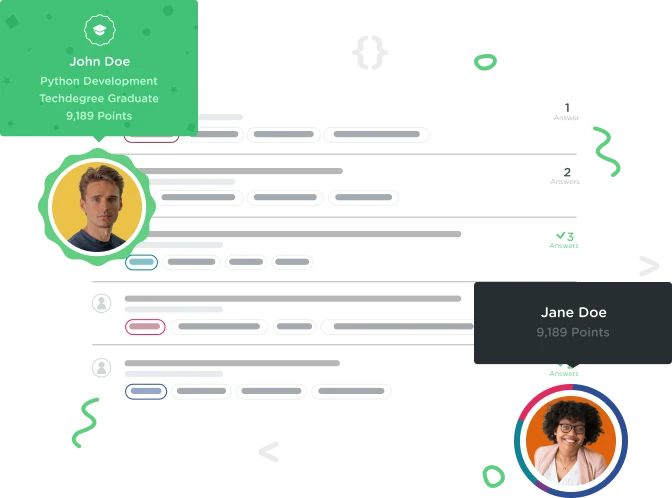

Jason Hill
Full Stack JavaScript Techdegree Student 11,399 PointsUp for review! Thanks!
So I learned a new Math.pow function to make my original more efficient, as I original had my math going in order, like radius * radius. I also just left it open for whatever input the user put in, but I did check to ensure it worked by using the parameters that were specified, and it is running correctly. Starting to learn how to look things up and refine stuff. Please check it out and let me know what you all think!
// 1. Attach this file geometry.js to the index.html file
// 2. Create a function that calculates the area of a rectangle.
// The function should accept the width and height as arguments
// and return the area of that rectangle.
// The area of a rectangle is the width * height
function areaRec( width, height){
var width = prompt("Enter width of rectangle:");
var height = prompt("Enter height of rectangle:");
console.log(width * height);
}
areaRec();
// 3. Create a function that calculates the volume of a rectangular prism.
// The function should accept the width, height and length as arguments
// and return the volume of that rectangular prism.
// The volume of a rectangular prism is the width * height * length
function volPrism(width, height, length){
var width = prompt("Enter the width of prism:");
var height = prompt("Enter the heigth of prism:");
var length = prompt("Enter the length of prism:");
console.log(width * height * length);
}
volPrism();
// 4. Create a function that calculates the area of a circle.
// The function should accept the radius of the circle as an argument
// and return the area of that circle.
// The area of a circle is the value of π * radius^2
function areaCir(pi, radius){
var radius = prompt("Enter the radius of circle:");
console.log(Math.PI * (radius * radius));
}
areaCir();
// 5. Create a function that calculates the volume of a sphere.
// The function should accept the radius of the sphere as an argument
// and return the volume.
// The volume of a circle is: 4/3 * π * radius^3
function volSphere(radius){
var radius = prompt("Enter the radius of the sphere:");
console.log(4 / 3 * Math.PI * (Math.pow(radius, 3)));
}
volSphere();
// 6. Use console.log to test each function and output to the JavaScript console
// Here are the values to test and the expected results
// -- Area of rectangle that is 5 wide and 22 tall: 110
// -- Volume of a rectangular prism that is 4.5 x 12.5 x 17.4: 978.7499999999999
// -- Area of a circle that with a radius of 7.2: 162.8601631620949
// -- Volume of a spehere with a radius of 7.2: 1563.4575663561109