Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial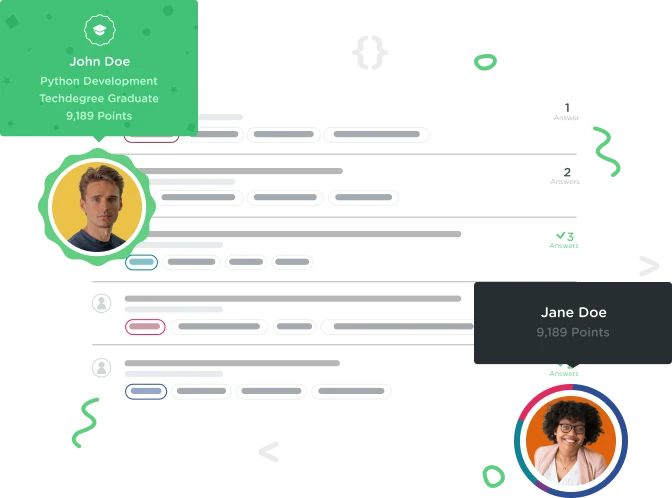
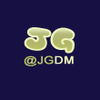
Jonathan Grieve
Treehouse Moderator 91,252 PointsUpdate project always adds a new project to the list.
Hi there,
I've been on this video now for a few days but I just can't seem to get past this minor but annoying bug. Every time I select a project to update it adds the project to the list rather than showing the updates.
Anyone who's done this course yet know what I might have missed? I can't see what I've missed and I'm out of ideas.
Github Repo: https://github.com/jg-digital-media/using_crud
<?php
//application functions
function get_project_list() {
//include connection database
include 'connection.php';
//error handling with try-catch
try {
//execute query and return result set.
return $db->query('SELECT project_id, title, category FROM projects');
} catch (Exception $e) {
echo "Error!: " . $e->getMessage() . "</br>";
return array();
}
}
function get_task_list($filter = null) {
//include connection database
include 'connection.php';
$sql = "SELECT tasks.*, projects.title as project FROM tasks"
. " JOIN projects ON tasks.project_id = projects.project_id";
$where = "";
if(is_array($filter)) {
switch($filter[0]) {
case 'project':
$where = ' WHERE projects.project_id = ?';
break;
case 'category':
$where = ' WHERE category = ?';
break;
case 'date':
$where = ' WHERE date >= ? AND date <= ?';
break;
}
}
$orderBy = ' ORDER BY date DESC';
if($filter) {
$orderBy = ' ORDER BY projects.title ASC, date DESC';
}
//error handling with try-catch
try {
//execute query and return result set.
$results = $db->prepare($sql . $where . $orderBy);
if (is_array($filter)) {
$results->bindValue(1, $filter[1]);
if($filter[0] == 'date') {
$results->bindValue(2, $filter[2], PDO::PARAM_STR);
}
}
$results->execute();
} catch (Exception $e) {
echo "Error!: " . $e->getMessage() . "</br>";
return array();
}
return $results->fetchAll(PDO::FETCH_ASSOC);
}
// method to add a new project to database
function get_project($project_id){
//include connection to the database.
include 'connection.php';
$sql = 'SELECT * FROM projects WHERE project_id = ?';
try {
$results = $db->prepare($sql);
$results->bindValue(1, $project_id, PDO::PARAM_INT);
$results->execute();
} catch(Exception $e) {
echo "Unable to retrieve query: ". $e->getMessage() . "<br />";
return false;
}
return $results->fetch();
}
// method to add a new project to database
function add_project($title, $category, $project_id = null){
//include connection to the database.
include 'connection.php';
if($project_id) {
$sql = 'UPDATE projects SET title = ?, category = ? WHERE project_id = ?';
} else {
$sql = 'INSERT INTO projects(title, category) VALUES(?, ?)';
}
try {
$results = $db->prepare($sql);
$results->bindValue(1, $title, PDO::PARAM_STR);
$results->bindValue(2, $category, PDO::PARAM_STR);
if($project_id) {
$results->bindValue(3, $project_id, PDO::PARAM_INT);
}
$results->execute();
} catch(Exception $e) {
echo "Unable to retrieve query: ". $e->getMessage() . "<br />";
return false;
}
return true;
}
// method to add a new project to database
function add_task($project_id, $title, $date, $time){
//include connection to the database.
include 'connection.php';
$sql = 'INSERT INTO tasks(project_id, title, date, time) VALUES(?, ?, ?, ?)';
try {
$results = $db->prepare($sql);
$results->bindValue(1, $project_id, PDO::PARAM_INT);
$results->bindValue(2, $title, PDO::PARAM_STR);
$results->bindValue(3, $date, PDO::PARAM_STR);
$results->bindValue(4, $time, PDO::PARAM_INT);
$results->execute();
} catch(Exception $e) {
echo "Unable to retrieve query: ". $e->getMessage() . "<br />";
return false;
}
return true;
}
require 'inc/functions.php';
$page = "reports";
$pageTitle = "Reports | Time Tracker";
$filter = 'all';
if(!empty($_GET['filter'])) {
//set filter variable equal to report form value
$filter = explode(':', filter_input(INPUT_GET, 'filter', FILTER_SANITIZE_STRING));
}
include 'inc/header.php';
?>
<div class="col-container page-container">
<div class="col col-70-md col-60-lg col-center">
<div class="col-container">
<h1 class='actions-header'>Displaying Report:
<?php if(!is_array($filter)) {
echo "All Tasks by Project";
} else {
echo ucwords($filter[0]) . " : ";
switch ($filter[0]) {
case 'project':
$project = get_project($filter[1]);
echo $project['title'];
break;
case 'category':
echo $filter[1];
break;
case 'date':
echo $filter[1] . " - " . $filter[2];
break;
}
} ?></h1>
<form class ="form-container form-report" action="reports.php" method="get">
<label for="filter">Filter:</label>
<select id="filter" name="filter">
<option value=''>Select a Project</option>
<optgroup label="Project">
<?php
foreach (get_project_list() as $option) {
echo '<option value="project:' . $option['project_id'] . '">';
echo $option['title'] . "</option>\n";
}
?>
</optgroup>
<optgroup label="Category">
<option value='category:Personal'>Personal</option>
<option value='category:Billable'>Billable</option>
<option value='category:Charity'>Charity</option>
</optgroup>
<optgroup label="Date">
<option value="date:<?php
echo date('m/d/Y', strtotime('-2 Sunday'));
echo ":";
echo date('m/d/Y', strtotime('-1 Saturday'));
?>">Last Week</option>
<option value="date: <?php
echo date('m/d/Y', strtotime('-2 Sunday'));
echo ":";
echo date('m/d/Y');
?>">This Week</option>
<option value="date: <?php
echo date('m/d/Y', strtotime('first day of last month'));
echo ":";
echo date('m/d/Y', strtotime('last day of last month'));
?>">Last Month</option>
<option value="date: <?php
echo date('m/d/Y', strtotime('first day of this month'));
echo ":";
echo date('m/d/Y');
?> ">Last This Month</option>
</optgroup>
</select>
<input class="button" type= "submit" value="Run Report" />
</form>
</div>
<div class="section page">
<div class="wrapper">
<table>
<?php
/*initialise starting total*/
$total = $project_id = $project_total = 0;
$tasks = get_task_list($filter);
foreach($tasks as $task) {
//loop through the tasks and add each item to the grand total
if($project_id != $task['project_id']) {
$project_id = $task['project_id'];
echo "<thead>\n";
echo "<tr>\n";
echo "<th>" . $task['project'] . "</th>\n";
echo "<th>Date</th>\n";
echo "<th>Time</th>\n";
echo "</tr>\n";
echo "</thead>\n";
}
$project_total += $task['time'];
$total += $task['time'];
echo '<tr>';
echo '<td>' . $task['title'] . '</td>'
. '<td>' . $task['date'] . '</td>'
. '<td>' . $task['time'] . '</td>';
echo '</tr>';
}
?>
<tr>
<th class='grand-total-label' colspan='2'>Grand Total</th>
<th class='grand-total-number'><?php echo $total; ?></th>
</tr>
</table>
</div>
</div>
</div>
</div>
<?php include "inc/footer.php"; ?>
<?php
require 'inc/functions.php';
$pageTitle = "Project | Time Tracker";
$page = "projects";
$title = $category ='';
//accept project id for project list
if(isset($_GET['id'])) {
list($project_id, $title, $category) = get_project(filter_input(INPUT_GET, 'id', FILTER_SANITIZE_NUMBER_INT));
}
if($_SERVER['REQUEST_METHOD'] == 'POST') {
//get values from form and filter input
$project_id = filter_input(INPUT_POST, 'id', FILTER_SANITIZE_NUMBER_INT);
$title = trim(filter_input(INPUT_POST, 'title', FILTER_SANITIZE_STRING));
$category = trim(filter_input(INPUT_POST, 'category', FILTER_SANITIZE_STRING));
if(empty($title) || empty($category)) {
//error message if empty fields
$error_message = 'Please fill in the required fields: Title and Category';
} else {
if(add_project($title, $category, $project_id)) {
header('Location: project_list.php');
exit;
} else {
$error_message = 'Could not add project. Check SQL Query';
}
}
}
include 'inc/header.php';
?>
<div class="section page">
<div class="col-container page-container">
<div class="col col-70-md col-60-lg col-center">
<h1 class="actions-header"><?php
if(!empty($project_id)) {
echo 'Update';
} else {
echo 'Add';
}
?> Project</h1>
<?php
if(isset($error_message)) {
echo "<p class='message'>$error_message</p>";
}
?>
<form class="form-container form-add" method="post" action="project.php">
<table>
<tr>
<th><label for="title">Title<span class="required">*</span></label></th>
<td><input type="text" id="title" name="title" value="<?php echo $title; ?>" /></td>
</tr>
<tr>
<th><label for="category">Category<span class="required">*</span></label></th>
<td><select id="category" name="category">
<option value="">Select One</option>
<option value="Billable"<?php
if($category == 'Billable') {
echo ' selected';
}
?>>Billable</option>
<option value="Charity"<?php
if($category == 'Charity') {
echo ' selected';
}
?>>Charity</option>
<option value="Personal"<?php
if($category == 'Personal') {
echo ' selected';
}
?>>Personal</option>
</select></td>
</tr>
</table>
<?php
if(!empty($project_id)) {
echo '<input type="hidden" name="id" value"' . $project_id . '" />';
}
?>
<input class="button button--primary button--topic-php" type="submit" value="Submit" />
</form>
</div>
</div>
</div>
<?php include "inc/footer.php"; ?>
1 Answer
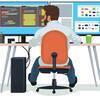
Veljo Palanen
29,914 PointsThe problem of adding a new project instead of updating the selected one seems to be on this line.
<?php
if(!empty($project_id)) {
echo '<input type="hidden" name="id" value"' . $project_id . '" />';
}
?>
To be working as UPDATE you need to change it to:
<?php
if (!empty($project_id)) {
echo "<input type='hidden' name='id' value='$project_id' />";
}
?>
When you download the files you can find it there also but the video code dosen't work as updateing.