Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial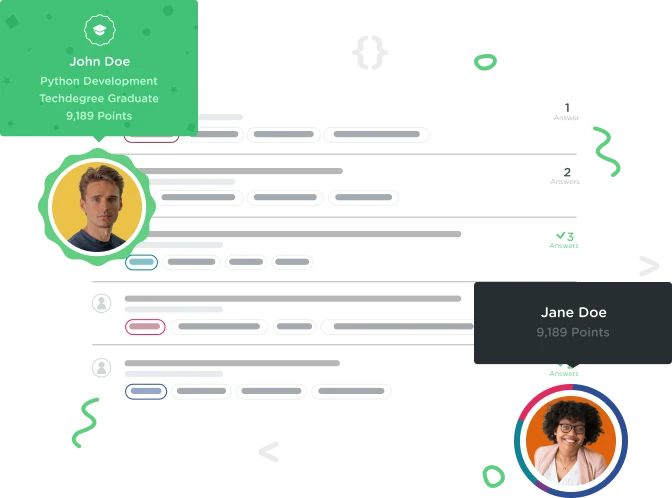

David Hautamaki
15,517 PointsupdateDisplay() null object reference
I am getting error messages "Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference" for my updateDisplay method in MainActivity. I tried to run debug to help. I can see my mCurrentWeather object populated with values, but they are not being set properly to the views for some reason in the updateDisplay method. How can I fix this null object reference? I'm thinking it might be issues with ButterKnife, since the syntax has changed recently, but I'm not sure. I've looked at similar questions, but no solutions so far have yielded a fix.
I am getting the following error:
FATAL EXCEPTION: main Process: dmtnt.com.weather, PID: 28262 java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference at dmtnt.com.weather.MainActivity.updateDisplay(MainActivity.java:95) at dmtnt.com.weather.MainActivity.access$200(MainActivity.java:29) at dmtnt.com.weather.MainActivity$1$1.run(MainActivity.java:77) at android.os.Handler.handleCallback(Handler.java:739) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:148) at android.app.ActivityThread.main(ActivityThread.java:5417) at java.lang.reflect.Method.invoke(Native Method) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Code below:
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@BindView(R.id.timeLabel) TextView mTimeLabel;
@BindView(R.id.tempLabel) TextView mTemperatureLabel;
@BindView(R.id.humidValue) TextView mHumidityValue;
@BindView(R.id.precipValue) TextView mPrecipValue;
@BindView(R.id.summaryLabel) TextView mSummaryLabel;
@BindView(R.id.iconImageView) ImageView mIconImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
String apiKey = "****************************************";
double latitude = 37.8267;
double longitude = -122.423;
String forecastURL = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastURL)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserAboutError();
}
} catch (IOException | JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else {
Toast.makeText(this, R.string.toast_conn_msg, Toast.LENGTH_LONG).show();
}
}
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemp() + "");
mTimeLabel.setText("At " + mCurrentWeather.getFormattedTime() + "it will be");
mHumidityValue.setText(mCurrentWeather.getHumidity() + "");
mPrecipValue.setText(mCurrentWeather.getPrecipChance() + "%");
mSummaryLabel.setText(mCurrentWeather.getSummary());
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemp(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogueFragment dialog = new AlertDialogueFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
1 Answer

Seth Kroger
56,413 PointsSince you're using version 8 of ButterKnife let's make sure it's set up as shown here:
https://teamtreehouse.com/community/more-deprecated-butterknife-code
David Hautamaki
15,517 PointsDavid Hautamaki
15,517 PointsYes, that was the issue. I had it setup like the previous versions.