Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial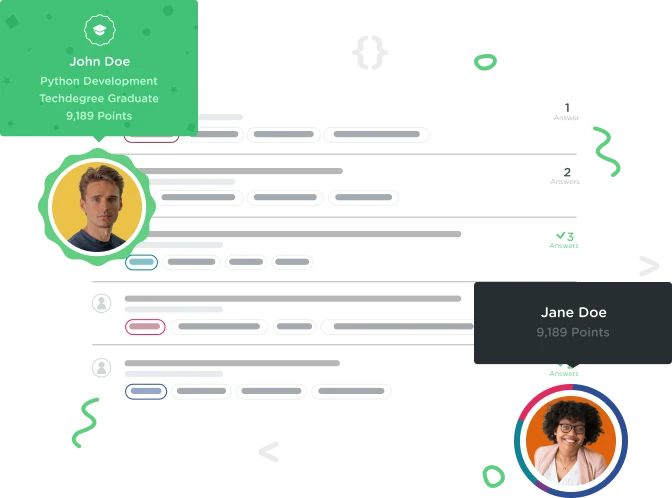

Killeon Patterson
18,528 Pointsupdating a cookie
Hello,
I'm attempting to have the changes made on the "settings" page to persist by setting a cookie. It's not working. The default.Theme cookie is created, but not updated. My page looks like the videos?
https://github.com/Kilosince/react-auth
import { createContext, useState, useEffect } from "react";
import Cookies from "js-cookie";
const ThemeContext = createContext(null);
export const ThemeProvider = (props) => {
const [isDarkMode, setIsDarkMode] = useState(false);
const [accentColor, setAccentColor] = useState('#63537d');
const [fontPercentage, setFontPercentage] = useState(100);
useEffect(() => {
if (isDarkMode) {
document.body.classList.add('dark');
} else {
document.body.classList.remove('dark');
}
document.body.style.fontSize = `${fontPercentage}%`;
const theme = {
isDarkMode,
accentColor,
fontPercentage
};
Cookies.set("defaultTheme", JSON.stringify(theme));
}, [isDarkMode, fontPercentage, accentColor]);
const toggleDarkMode = () => {
setIsDarkMode(currentMode => !currentMode);
}
return (
<ThemeContext.Provider value={{
accentColor,
isDarkMode,
fontPercentage,
actions: {
toggleDarkMode,
updateAccentColor: setAccentColor,
updateFontPercentage: setFontPercentage
}
}}>
{props.children}
</ThemeContext.Provider>
);
}
export default ThemeContext;
```JS
1 Answer
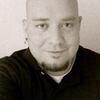
Brian Jensen
Treehouse StaffHiya Killeon Patterson
Your code works perfectly for me (which appears to be the code leading up to the 3-minute 40-second video mark), and the cookie updates when the cookies panel is refreshed. What browser are you using? A browser setting or extension may block the cookie from being updated.
I would recommend trying a different browser as a test. Then, if you experience the same issue in another browser, try the fully finished code of the video that utilizes the defaultTheme
object to handle each piece of state:
import { createContext, useState, useEffect } from "react";
import Cookies from "js-cookie";
const ThemeContext = createContext(null);
export const ThemeProvider = (props) => {
const cookie = Cookies.get("defaultTheme");
const defaultTheme = cookie ? JSON.parse(cookie) : {
isDarkMode: false,
accentColor: '#63537d',
fontPercentage: 100
}
const [isDarkMode, setIsDarkMode] = useState(defaultTheme.isDarkMode);
const [accentColor, setAccentColor] = useState(defaultTheme.accentColor);
const [fontPercentage, setFontPercentage] = useState(defaultTheme.fontPercentage);
useEffect(() => {
if (isDarkMode) {
document.body.classList.add('dark');
} else {
document.body.classList.remove('dark');
}
document.body.style.fontSize = `${fontPercentage}%`;
const theme = {
isDarkMode,
accentColor,
fontPercentage
};
Cookies.set("defaultTheme", JSON.stringify(theme));
}, [isDarkMode, fontPercentage, accentColor]);
const toggleDarkMode = () => {
setIsDarkMode(currentMode => !currentMode);
}
return (
<ThemeContext.Provider value={{
accentColor,
isDarkMode,
fontPercentage,
actions: {
toggleDarkMode,
updateAccentColor: setAccentColor,
updateFontPercentage: setFontPercentage
}
}}>
{props.children}
</ThemeContext.Provider>
);
}
export default ThemeContext;
Killeon Patterson
18,528 PointsKilleon Patterson
18,528 PointsThank you for your response. Yeah, it looked like I had the same code as the video. I tried it in explorer and chrome and it wouldn't update. I copied your code (which looked similar to mine) and it worked. Thank you for help and reply.