Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial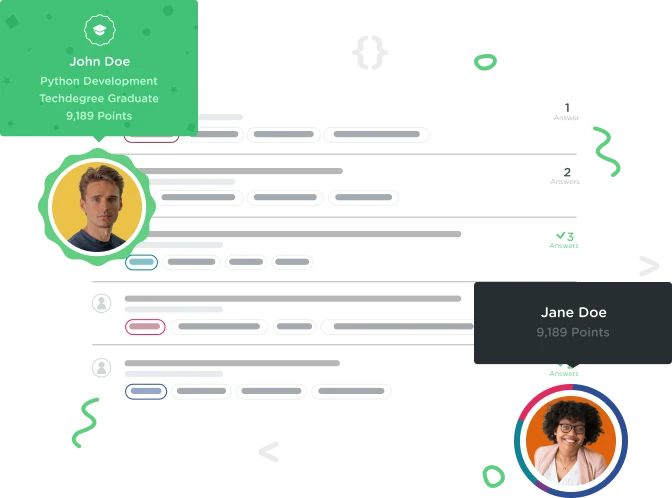

Samuel Trejo
4,563 PointsUpdating Buttons Challenge by Removing/Readding
I saw that most people opted towards hiding and revealing the buttons, so wanted to share my solution in which I clear all the buttons if there are any then reassign them after an event takes place that moves the list items.
Not sure, but it's possible hiding and revealing buttons as they move might be a better solution and probably faster? Maybe somebody can answer that, but I it did this way because I thought it would be more challenging.
Hope this helps somebody! Happy coding! Please provide feedback or recommendations if you have any.
const list = document.querySelector("ul.list");
const input = document.querySelector("input");
const addButton = document.querySelector(".add");
updateList()
//*------------------ Creates Buttons ------------------*//
function addRemoveBtn(li){
let remove = document.createElement("button");
remove.className = "remove";
remove.textContent = "Remove";
li.appendChild(remove);
}
function addUpBtn(li){
let up = document.createElement("button");
up.className = "up";
up.textContent = "Up";
li.appendChild(up);
}
function addDownBtn(li){
let down = document.createElement("button");
down.className = "down";
down.textContent = "Down";
li.appendChild(down);
}
//*------------------ Removes Buttons ------------------*//
//removes all current buttons from list items to prepare for reassignment
function removeLiBtns(li){
let remove = li.querySelector(".remove")
let up = li.querySelector(".up")
let down = li.querySelector(".down")
if(remove !== null){
li.removeChild(remove)
}
if(up !== null){
li.removeChild(up)
}
if(down !== null){
li.removeChild(down)
}
}
//*------------------ Attaches Buttons ------------------*//
//attaches buttons based on criteria below,
function attachLiBtns(li){
//if no list items before or after target list item, only remove button is added
if(li.previousElementSibling === null && li.nextElementSibling === null){
addRemoveBtn(li)
//if no list item before target list item, only down and remove buttons are added
}else if(li.previousElementSibling === null){
addDownBtn(li)
addRemoveBtn(li)
//if no list item after target list item, only up and remove buttons are added
}else if(li.nextElementSibling === null){
addUpBtn(li)
addRemoveBtn(li)
//if list items before and after target list item, all buttons are added
}else{
addUpBtn(li)
addDownBtn(li)
addRemoveBtn(li)
}
}
//*--------------------- Updates List ---------------------*//
//updates list by removing and re-adding the buttons
function updateList(){
let listChildren = list.children
for(i = 0; i < listChildren.length; i++){
removeLiBtns(listChildren[i])
attachLiBtns(listChildren[i])
}
}
//*--------------- Event Listener Functions ---------------*//
addButton.addEventListener("click", () => {
let li = document.createElement("li")
li.textContent = input.value
list.appendChild(li)
//calls updateList function to update the list
updateList()
input.value = ""
})
list.addEventListener("click", (event) => {
if(event.target.className === "remove"){
let li = event.target.parentNode
list.removeChild(li)
//calls updateList function to update the list
updateList()
}
if(event.target.className === "up"){
let li = event.target.parentNode
let prevLi = li.previousElementSibling
if(prevLi){
list.insertBefore(li, prevLi)
//calls updateList function to update the list
updateList()
}
}
if(event.target.className === "down"){
let li = event.target.parentNode
let nextLi = li.nextElementSibling
list.insertBefore(nextLi, li)
//calls updateList function to update the list
updateList()
}
})