Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial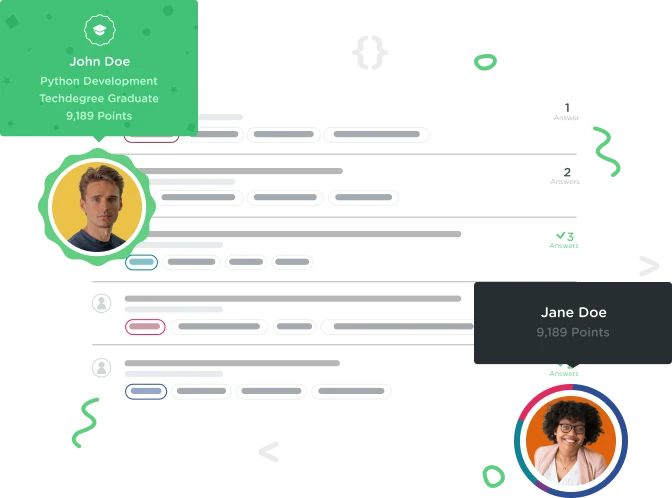

Will Beasley
6,020 Pointsupdating timezone from hill_valley to paris
This makes no sense. Not sure what's going on but here's what I've got:
import datetime
naive = datetime.datetime(2015, 10, 21, 4, 29) pacific = datetime.timezone(datetime.timedelta(hours = -8)) europe = datetime.timezone(datetime.timedelta(hours = 1)) hill_valley = naive.replace(tzinfo = pacific) paris = hill_valley.replace(tzinfo = europe)
It's saying that paris and hill_valley have the same timezone and I can't figure out why. To my knowledge primitive data types are immutable in python so paris = hill_valley.replace(...) would create a new var in memory not overwrite an existing vars values so any idea what's up? Thanks!
import datetime
naive = datetime.datetime(2015, 10, 21, 4, 29)
pacific = datetime.timezone(datetime.timedelta(hours = -8))
europe = datetime.timezone(datetime.timedelta(hours = 1))
hill_valley = naive.replace(tzinfo = pacific)
paris = hill_valley.replace(tzinfo = europe)
2 Answers

Will Beasley
6,020 PointsFigured it out, apparently instead of using the replace() method you call: paris = hill_valley.astimezone(europe)
I would like to know why astimezone and replace aren't interchangeable since the default value is probably none so why can't astimezone be used for hill_valley and why can't replace be used for paris? I'm really asking, I'd be interested to know the nuances.
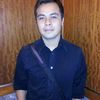
Jose Luis Lopez
19,179 Pointsin this question I used
you have to define europe/paris timezone too UTC =+ 01:00 paris = hill_valley.astimezone(you need a timezone here)
Cristian Altin
12,165 PointsCristian Altin
12,165 PointsI tested your code using replace and it's giving this output:
So the two timezones are different but the time is the same. I'd say you are only replacing the timezone but keeping the time.
Using astimezone the output is the following:
As you can see astimezone also converted the time from hill_valley to paris.
This is why you cannot use them interchangeably.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherBecause
.replace()
just changes the timezone's value, it doesn't move thedatetime
to the new timezone.astimezone()
moves thedatetime
to be the correct value for the new timezone.Cristian Altin
12,165 PointsCristian Altin
12,165 PointsAs also Kenneth said. Consider that the two exist because at times you might need to keep the hours and minutes and adapt them to another timezone while other times you just need to convert them to another timezone.
Suppose for example that you made a script to handle party events that start in the US, Europe and Asia all locally at 8PM. You will use replace() to keep the date and hour but the timezone will change. The rest is DRY.
On the other hand suppose you need to know what was your local time when someone entered your secret facility on the other side of the world while you didn't notice. Then you will have to use astimezone() to find out you were having a siesta early in the afternoon when someone entered the facility during the night. Knowing the time at the facility doesn't help you so replace() would not be the right choice here.
And no, I don't have any secret facility...maybe...hmmm no, definitely. ;-)