Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial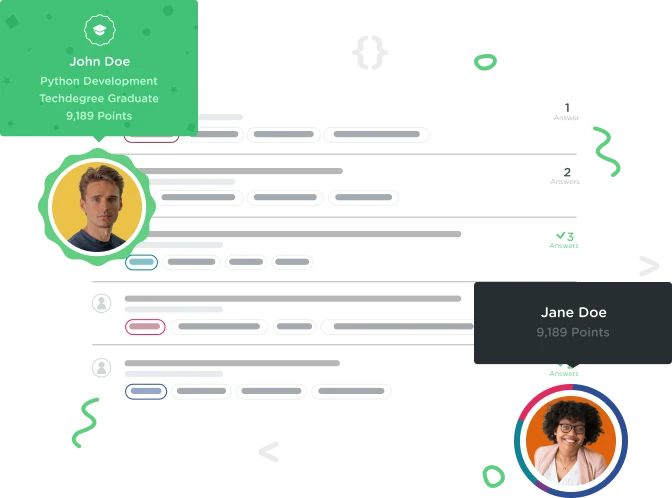
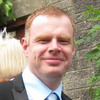
Rob Randell
13,809 PointsUpdating UILabel with Delegate
Hi guys, I'm trying to use a simple delegate to update a label with a string value from another class but the label doesn't update and it appears that the delegate isn't responding to the selector method. I've watched the Deep Dive video regarding delegates and this used a tableview to add an additional row using values from another class. Obviously, I'm not understanding something and missing something really obvious.
This is using a storyboard with a segue using a modal transition to open the other view controller.
Here is what I have:
ViewController.h
#import <UIKit/UIKit.h>
#import "RJRBarCodeViewController.h"
@interface RJRViewController : UIViewController <PassUserCodeDelegate> {
IBOutlet UILabel *codeLabel;
}
@property (nonatomic) NSString *amendedString;
- (IBAction)openScanner:(id)sender;
@end
ViewController.m
#import "RJRViewController.h"
@interface RJRViewController ()
@end
@implementation RJRViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (IBAction)openScanner:(id)sender {
RJRBarCodeViewController *scannerClass = [[RJRBarCodeViewController alloc] init];
scannerClass.codeDelegate = self;
}
- (void)userCode:(NSString *)str {
codeLabel.text = str;
NSLog(@"New String: %@", str);
}
@end
RJRBarCodeViewController.h
#import <UIKit/UIKit.h>
@protocol PassUserCodeDelegate <NSObject>
- (void)userCode:(NSString *)str;
@end
@interface RJRBarCodeViewController : UIViewController {
__weak id <PassUserCodeDelegate> codeDelegate;
}
@property (nonatomic, weak) id <PassUserCodeDelegate> codeDelegate;
@property (nonatomic, weak) NSString *codeString;
- (IBAction)done:(id)sender;
@end
RJRBarCodeViewController.m
#import "RJRBarCodeViewController.h"
@interface RJRBarCodeViewController ()
@end
@implementation RJRBarCodeViewController
@synthesize codeDelegate;
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
self.codeString = @"empty string";
}
- (IBAction)done:(id)sender {
if([codeDelegate respondsToSelector:@selector(userCode:)]) {
[self.codeDelegate userCode:self.codeString];
NSLog(@"Delegate Method Called... with %@", self.codeString);
}
[self dismissViewControllerAnimated:YES completion:nil];
}
2 Answers
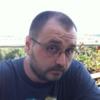
Robert Bojor
Courses Plus Student 29,439 PointsHi Rob,
You will need to use prepareForSegue:sender: method in order access the instance of the destination view controller that is going to open.
In the openScanner: method you are calling you are actually creating a new instance of the destination view controller and the segue you've set up is using another instance.
Here's a link to a project that is using a delegate method to pass data from the second to the first view controller: https://dl.dropboxusercontent.com/u/7220030/DelegateTest.zip
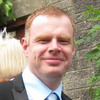
Rob Randell
13,809 PointsThanks Rob. I thought that was the case that it was creating a new instance instead of accessing the original instance. I'll take a look at the Test Project and see if that helps me make any head way.
EDIT: Thanks Rob, I've managed to update the label successfully.
Cheers.
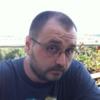
Robert Bojor
Courses Plus Student 29,439 PointsNo problem, happy it helped :)