Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial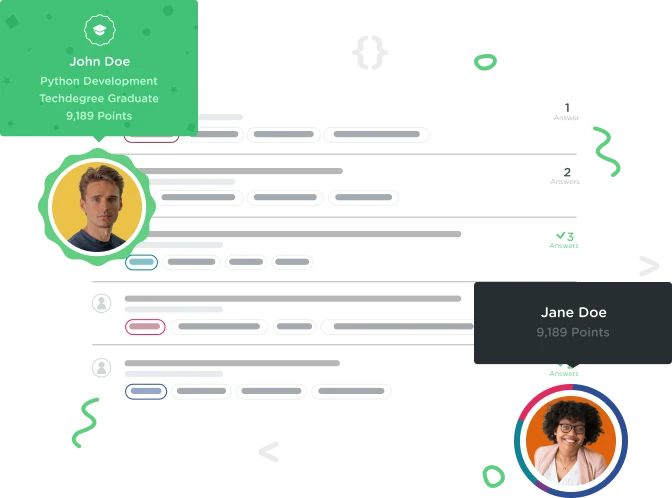

Y B
14,136 PointsUpgrading React Components to ES6
I'm currently on the React and Redux course where we are upgrading React components to ES6 where we are using arrow functions for: (1) SFC's and (2) in Class based components methods
Is there any difference/ benefit to using arrow functions for 1? eg
function Player(props) {}
vs
Player = props => {}
For 2, this has the benefit of not having to explicitly bind this, as they use the parent's scope. However I thought using arrow functions leaves an undefined function in the stack making it harder to debug - is this true in these use cases? I had instead used an alias in the constructor to bind instead. The use of an arrow function seems a bit visually noisy vs just using a normal (unbound method). Is the arrow syntax preferred now?
eg
class AddPlayerForm extends React.Component {
constructor(props) {
super(props);
this.state = {name: '',};
// this.state.name = this.state.name.bind(this);
this.onNameChange = this.onNameChange.bind(this)
}
onNameChange(e){
this.setState({name: e.target.value});
}
// Note difference style to binding this vs onNameChange
onSubmit = (e) => {
e.preventDefault();
this.props.onAdd(this.state.name);
this.setState({name: ""});
}
render() {
return (
<div className="add-player-form">
<form onSubmit={this.onSubmit}>
<input type="text" value={this.state.name} onChange={this.onNameChange}/>
<input type="submit" value="Add Player"/>
</form>
</div>
);
}
}
AddPlayerForm.propTypes = {
onAdd: PropTypes.func.isRequired,
};
Additionally in the video we put state just inside the class as
class AddPlayerForm extends Component {
state = { name: ""}
....}
rather than in the constructor as I had. What's the benefit on one approach over the other?