Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial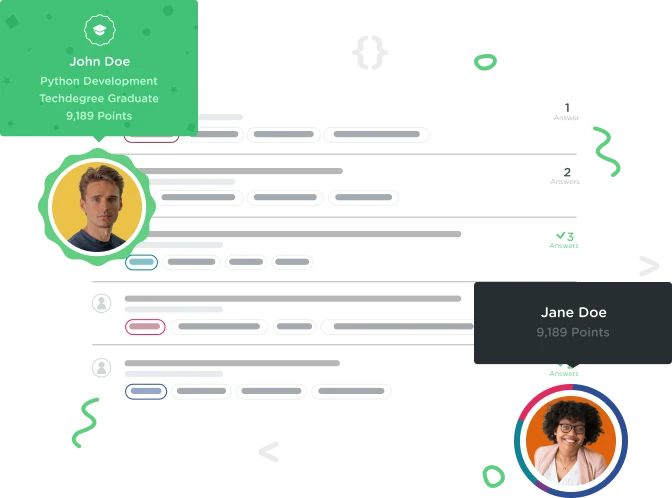

FRED NUPUKU TAMBUA
173 Pointsupload msword file with php
Helo please i am building a website and i need users to be able to submit an msword file with file name and description and display it on another page.I am using php .Thanks.I have got a database with table that has 3 fields; file name, file description and file
7 Answers

Lee Chavers
22,506 PointsFirst things first Fred, make sure you're form has this attribute
<form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post"
enctype="multipart/form-data"></form>
Now for the actual upload process (in php) here's what I do
// functions for handling a few things during the file upload process
// cleans input
function cleanname($input) {
return strtolower(headline($input));
}
// find the basename of a file
function findbase($filename) {
$base = explode(".", $filename);
$count = (count($base)-1);
$i = 0;
while($i < $count) {
$returnbase=$returnbase . $base[$i] . ".";
$i++;
}
$returnbase = rtrim($returnbase, '.');
return cleanname($returnbase); // cleans the file basename
}
// finds the extension of a file
function findext($filename) {
$base = explode(".", $filename);
$count = count($base)-1;
$ext = $count;
return ".".$base[$ext];
}
// format a headline for url
function headline($headline) {
// strip out bad characters
$headline = str_replace("'s", "s", $headline);
$headline = str_replace("'d", "d", $headline);
$headline = str_replace("'t", "t", $headline);
$headline = str_replace("'ll", "ll", $headline);
$headline = str_replace("'", "", $headline);
$headline = str_replace(" ", "-", $headline);
$headline = str_replace("&", "-", $headline);
$headline = str_replace("–", "-", $headline);
$headline = str_replace("’", "-", $headline);
$headline = str_replace("”", "-", $headline);
$headline = str_replace("‘", "-", $headline);
$headline = str_replace("“", "-", $headline);
$headline = str_replace(",", "", $headline);
$headline = str_replace(";", "", $headline);
$headline = str_replace(":", "", $headline);
$headline = str_replace("!", "", $headline);
$headline = str_replace(".", "", $headline);
$headline = str_replace("?", "-", $headline);
$headline = str_replace("&", "-", $headline);
$headline = str_replace("---", "-", $headline);
$headline = str_replace("--", "-", $headline);
$headline = str_replace(array('!', '\\', '*', '?', '(', ')'), '', $headline);
$headline = preg_replace('/[^A-Za-z0-9_.-]/', '', $headline);
$headline = stripslashes($headline);
$headline = strtolower($headline);
return $headline;
}
// config for our upload
$path = "../files/";
$tempname = $_FILES['form']['tmp_name'];
$filename = $_FILES['form']['name'];
$newfilename = findbase($filename).findext($filename);
// make sure the same file isn't in our folder
if(file_exists($path.$filename)) {
$newfilename = findbase($filename).rand(1,5555).findext($filename);
}
// upload our file
// if our file was unable to be put in place, show an error message
if(!copy($tempname, $path.$newfilename)) {
$errors['form'] = "There was an error uploading the form. Please try again.";
print_r($_FILES);
// file was copied over successfully
} else {
// make sure our file has the proper permissions
chmod($path.$newfilename, 0644);
upload information about our file into the database
$sql = "INSERT into files set name='$name', filename='$newfilename', last_updated=NOW()";
$result = mysql_query($sql);
}
I use a couple of functions to find the base and extension of our file that was uploaded. I've included the two functions above the processing code. They need to be kept there or moved to a file that contains all your functions.
Keep in mind that you'll probably need 777 permissions on the folder that you are wanting to upload files to.

FRED NUPUKU TAMBUA
173 PointsThank you very much. Here is my html form;
<form action="upload.php" method="post" enctype="multipart/form-data"> <ul><form action="upload.php" method="post" enctype="multipart/form-data"> <ul> <li> Project Name<br><input type="text" name="project_name"> </li>
<li>
Project Description<br><textarea name="project_desc" rows="3" cols="30"></textarea>
</li>
<li>
Project Documentation
//<input type="hidden" name="MAX_FILE_SIZE" value="2000000"><br>
<input name="file" type="file" id="file">
<p><em>MsWord Document Only</em></p>
</li>
<li>
<input type="submit" name="submit" value="Add Project">
</li>
</ul>
</form> <li> Project Name<br><input type="text" name="project_name"> </li>
<li>
Project Description<br><textarea name="project_desc" rows="3" cols="30"></textarea>
</li>
<li>
Project Documentation
//<input type="hidden" name="MAX_FILE_SIZE" value="2000000"><br>
<input name="file" type="file" id="file">
<p><em>MsWord Document Only</em></p>
</li>
<li>
<input type="submit" name="submit" value="Add Project">
</li>
</ul>
</form>
I actually need to store the filename and file description in a database and then the msword file in a folder, then display them on a different page such that the Msword file can be downloaded.
Thank you

Lee Chavers
22,506 PointsFred, where is your <form>
in your code you provided above?

FRED NUPUKU TAMBUA
173 Pointscode <form action="upload.php" method="post" enctype="multipart/form-data"> <ul> <li> Project Name<br><input type="text" name="project_name"> </li>
<li>
Project Description<br><textarea name="project_desc" rows="3" cols="30"></textarea>
</li>
<li>
Project Documentation
//<input type="hidden" name="MAX_FILE_SIZE" value="2000000"><br>
<input name="file" type="file" id="file">
<p><em>MsWord Document Only</em></p>
</li>
<li>
<input type="submit" name="submit" value="Add Project">
</li>
</ul>
</form>

FRED NUPUKU TAMBUA
173 Pointsits being stripped out, don't know why it's got an opening and closing form tag with the method="post" enctype="multipart/form-data

Lee Chavers
22,506 PointsOkay, just wanted to make sure you had that. In the php code I gave you'll need to replace this two guys. I changed their respective fields from my code to match what you've provided.
$tempname = $_FILES['file']['tmp_name'];
$filename = $_FILES['file']['name'];

Lee Chavers
22,506 PointsJust wanted to follow up and see if this worked for you?