Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial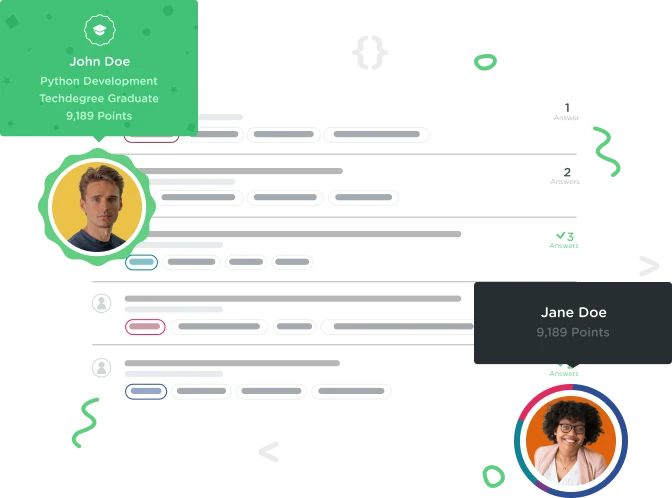
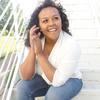
Enzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsUppercase and Lowercase with Slices
Here's the question:
I need you to create a new function for me. This one will be named sillycase and it'll take a single string as an argument. sillycase should return the same string but the first half should be lowercased and the second half should be uppercased. For example, with the string "Treehouse", sillycase would return "treeHOUSE". Don't worry about rounding your halves, but remember that indexes should be integers. You'll want to use the int() function or integer division, //.
I am quite lost, and don't know where to start. PLEASE answer step-by-step; I am new at this! Thanks!
def sillycase(single_string):
sillycase.index(single_string)
2 Answers
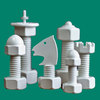
Steven Parker
230,274 PointsOne approach would involve these steps:
- using the word length, compute what half of it would be (make sure the result is integer)
- using that value, make a slice of the first part of the word
- similarly, make a slice of the last part
- convert the first part to lower case
- convert the last part to upper case
- use concatenation to join the parts together
- return the joined word

Yan Kozlovskiy
30,427 PointsProgramming is hard and very confusing at first. I can't speak for others, but for me it was and still is. I'm learning popular algorithms and C right now, which should help me become a better programmer. Some people get it a lot better than others. I still don't get a lot of things. It's hard work, but don't get discouraged. Personally, I'm now at the point where I can create basic things (create a basic frontend and a backend with authentication and use external APIs and plugins to load data) and can read guides and the documentation to come up with a solution. You'll get there, we're all still struggling with you because all of us are striving to understand more and improve the quality of our work.
Python is a bit strange in the sense that the slicing feels unintuitive. For whatever reason, you can't do -1 to access the last index of a string. The -1 will give you the second to last character. Therefore, my solution consists of using the "len" function and store the result in a variable to access that value later in the function.
I've been programming a lot in JavaScript lately, so forgive me if this looks like JS, LOL I haven't touched python in many months. In JavaScript, you do a lot of variable assignments and reassignments and this is where my head is stuck at the moment. Here's a solution I coded to solve what you're trying to do. It's not the nicest looking function in the world, but it works.
import math
def sillycase(single_string):
total_length = len(single_string)
second_half_first_index = math.floor(total_length / 2)
left = single_string[0:first_half_last_index]
right = single_string[second_half_first_index:total_length]
print(left.lower() + right.upper())
sillycase("treehouse")
Here's a breakdown of the code:
Line 1: import math
You need to import the builtin math module to access specific functions used in our custom function. In this case, we're using math.floor to get rid of the decimal and keep the whole number.
Line 3: def sillycase(single_string):
The def keyword tells the interpreter to create a new function. The function "sillycase" contains one parameter called "single_string" which will store a single argument that is being passed in, each time the function gets called.
Line 4: total_length = len(single_string)
Here, the len function returns a number that contains the total number of items in the string. The count starts at 1, unlike arrays where the count starts at 0. So, if our string is "treehouse", len("treehouse") returns 9.
Line 5: second_half_first_index = math.floor(total_length / 2)
This line creates a variable called "second_half_first_index" and stores the result of the following expression: "math.floor(total_length / 2)". Here math.floor from the math module is used to remove the decimal value after dividing the total length of the string by 2, or half. This is to get the first index of the character where the second half of the string starts. This is useful to know because we want to know where to start uppercasing the string and where the boundary ends for lowercasing the string.
Line 6: left = single_string[0:second_half_first_index]
The variable name "left" describes the left side of the string, or the side that is to be lowercased. The variable contains a slice of the parameter "single_string" and it grabs the first index of the string denoted by the 0 and it finishes at the e. The value stored in this variable is "tree" because the way slicing works, in Python, is that the first value is the 0 index. The second value for slicing is starting the count from 1. So, in this case we have 4 as the last value. Our parameter has "treehouse" stored in it. Therefore, single_string[0:4] returns "tree" because 0 = "t" and 4 = "e".
Line 7: right = single_string[second_half_first_index:total_length]
The variable name "right" describes the right side of the srting, or the side that needs to be upper cased. This function starts at the index position 4, which is "h" and ends at the position of the total length of the string as defined by the "total_length" variable which stores the integer "9".
Line 8: print(left.lower() + right.upper())
The print function outputs data to the screen. Here we're using concatenation to display the result. We combine the variable "left" and pass it into a standard builtin function called "lower()" to lowercase all the characters. We do the same thing for the variable "right", except we're using the the "upper()" function to uppercase all the characters inside of the variable. The result of print(left.lower() + right.upper()) outputs "treeHOUSE" as the result.
Line 10: sillycase("treehouse")
This line calls our custom function named "sillycase" and uses the string "treehouse" as an argument.
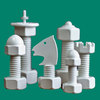
Steven Parker
230,274 Points For the challenge, you only define the function. You don't call it.