Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial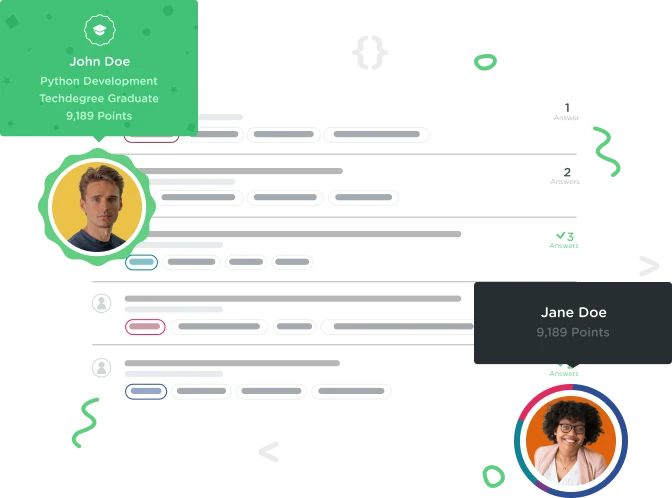

Ibrahim Nura
Courses Plus Student 1,404 PointsUrgent I need help
Cant use getters method
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.id.passengersEditText);
// Add your code here!
mSpaceship=new Spaceship("FIREFLY");
public Spaceship getSpaceship(){
return mSpaceship;
}
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type) {
mType = type;
}
}
1 Answer

Keli'i Martin
8,227 PointsI'm not sure which part of the challenge you are on, but I'll assume you are on Task 2, since you seem to already have answered Task 1 correctly. So Task 2 is asking you to update the TextView with the spaceship's type. I'm not exactly sure what you're trying to do with the function in your activity, but go ahead and remove that.
What you need to do is set the text of the TextView with the spaceship's type, using the getter for the type. First, in order to set a TextView's text, you need to call its setText()
method. So in this case:
mTypeLabel.setText()
The parameter for this method is the text you want to display in the TextView, which in this case is the spaceship's type. You will get that by using the getter, like this:
mSpaceship.getType()
Putting it all together, this is the line you need to add to accomplish Task 2:
mTypeLabel.setText(mSpaceship.getType());
Hope this helps!