Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial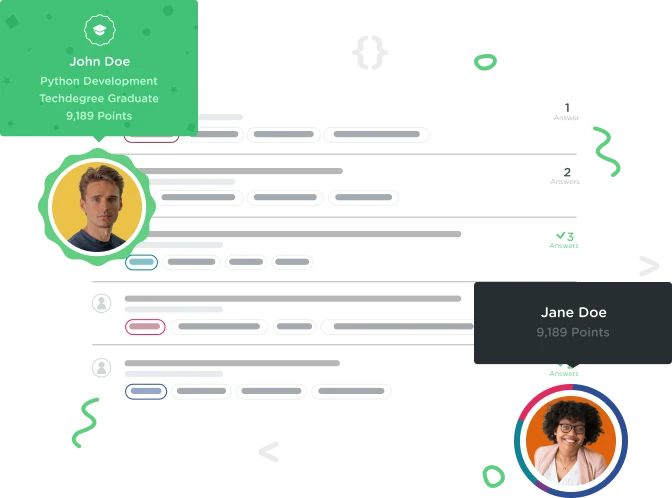
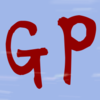
Dallas Smith
2,563 PointsUri parsing problem (code challenge)
I am trying to do the code challenge that provides a string array with urls and parse it similar to what is done in the video.
Since this challenge doesn't use JSON I'm somewhat confused on how to set it up to actually get the position of the tap and set the string equal to that url. Here is the code in its entirety. Any help is appreciated :)
protected String[] mUrls = { "http://www.teamtreehouse.com", "http://developer.android.com", "http://www.github.com" };
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_custom_list);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, mUrls);
setListAdapter(adapter);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
// Add code here!
super.onListItemClick(l, v, position, id);
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(mUrls));
startActivity(intent);
}
}
1 Answer

Daniel Hartin
18,106 PointsHi Dallas,
Your code only has 1 little problem, you need to specify which element of the array is to be used as the String variable. You can do this by taking advantage of the position parameter passed into the method call.
Use the code below:
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(mUrls[position]));
startActivity(intent);
}
Hope this helps Daniel