Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial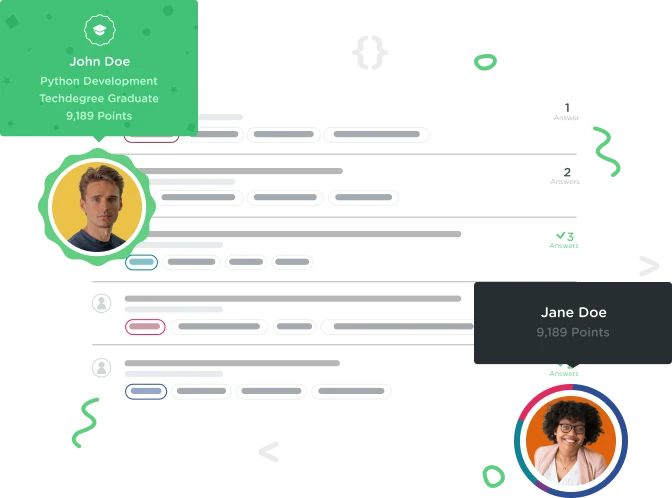
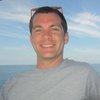
Jason Law
15,254 PointsUrllib2 not supported in python 3. How can the import script be adjusted to do the same thing without urllib2?
I would like to download the data directly, but I am having issue because I am using Python 3 instead of Python 2. Specifically, my code is breaking because urlopen(ties) does not return json, but instead returns a HTTPResponse class. How can I adjust this to make it work with Python 3?
from urllib.request import urlopen
import json
import pandas as pd
my_api_key = "xxxxxxx"
url = "http://api.shopstyle.com/api/v2/"
ties = "{}products?pid={}&cat=mens-ties&limit=100".format(url, my_api_key)
jsonResponse = urlopen(ties)
print(type(jsonResponse)) # returns <class 'http.client.HTTPResponse'>
data = json.load(jsonResponse) # this errors because jsonResponse is not json
Jason Law
15,254 PointsJason Law
15,254 PointsI figured it out. The read() method and decode() method were required to return the http response as json. This is the script that worked for me in python 3.