Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial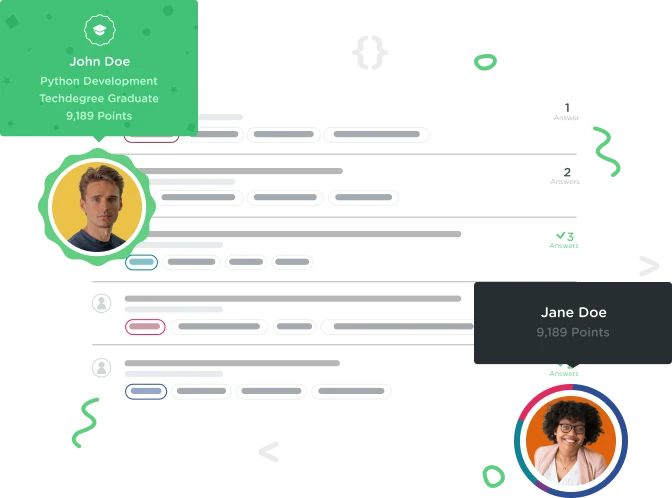
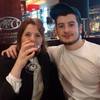
Oliver Sewell
16,108 PointsUse a for in loop to log each of the property names of the shanghai object to the console. what have i done wrong? T_T
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for (prop in shanghai) {
console.log(shanghai.[prop]);
}
5 Answers

Chase Marchione
155,055 PointsHi Oliver,
For task 1:
1) We want to use the 'var' keyword when we declare our key. 2) Simply supplying the key as a parameter to the log method does the trick (no need for any kind of Object.key syntax, in this case) for task 1, because we are only asked to include keys, and not values. The compiler knows which items we're referencing. In task 2, we'll do it a little differently, though.
Task 1 sample code:
for (var prop in shanghai) {
console.log(prop);
}
For task 2:
The challenge asks us to log out both keys and values now, but also in a specific format (e.g. "population: 1434e6"). You are on the right track in terms of retrieving the data, but the '.' between shanghai and [prop] is not necessary.
Task 2 sample code (make sure you delete your task 1 code when you do task 2):
for (var prop in shanghai) {
console.log(prop, ': ', shanghai[prop]);
}
Hope this helps!

Ali Al Hudari
9,876 Pointsvar shanghai = { population: 14.35e6, longitude: '31.2000 N', latitude: '121.5000 E', country: 'CHN' }; for(var prop in shanghai){ console.log(prop); }
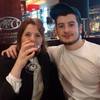
Oliver Sewell
16,108 Pointsyes thankyou for the detailed explanation ! best answer received so far! xD
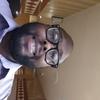
darrin allen
Full Stack JavaScript Techdegree Student 1,798 Pointsfor (var joke in shanghai) { console.log(joke, shanghai[joke]); };
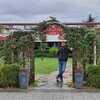
Hassan Al Manawy
24,859 PointsHey,
Aside from the challenge, this code worked perfectly fine in my own text editor "Atom" without the var keyword.
Still not sure why it is needed here, but anyway I guess declaring variables is one of JS best practices. Also, that is how we have been taught to write the "for" loops in the previous section: for (var i = 0; i < 10; i++) .
Happy coding, everyone.
Nicholas Lee
12,474 PointsNicholas Lee
12,474 PointsIn the video prior to this challenge it works without having to instantiate the 'var' variable inside the ' for in' loop. I'm wondering, why is this?
https://teamtreehouse.com/library/javascript-loops-arrays-and-objects/tracking-data-using-objects/using-for-in-to-loop-through-an-objects-properties,
Freyja Nash
2,781 PointsFreyja Nash
2,781 PointsI'm a little confused why we need to do var prop when the video beforehand does not use var prop. Using just 'prop' prints the exact same thing to the console as using 'var prop'.
Kate C
13,589 PointsKate C
13,589 Pointsi have same question in 2020! why var is needed ? I was thinking what went wrong with my code for a while.