Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial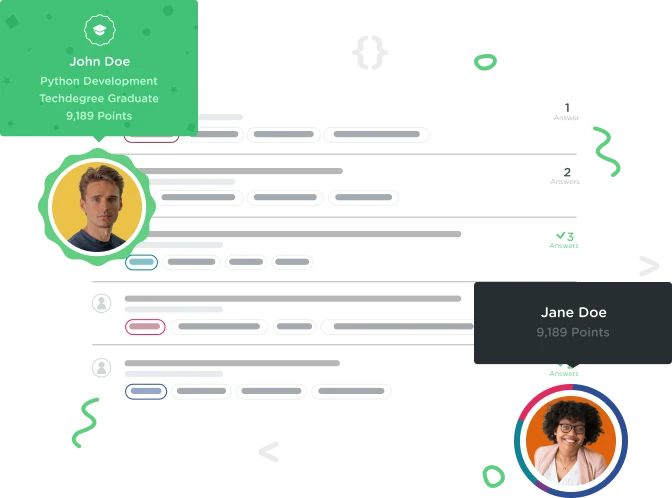
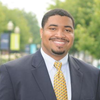
Henry Morrow
19,425 PointsUse addEventListener to bind a keyup event to the enter key.
Is there a way to use addEventListener to bind the addTask to the enter key and same to bind the save button while in edit mode for the todos? Ideally I would like the let the user add a new to do just by pressing enter as well as using the click event.
2 Answers
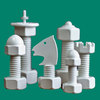
Steven Parker
231,269 PointsSure, Henry, that's reasonably easy. But you probably want to bind "keypress" instead of "keyup". And you'll need an intermediate handler to make sure to only add a task when the key pressed is Enter.
Something like this:
taskInput.addEventListener("keypress", keyPressed); // bind to taskInput, not addButton
function keyPressed(k) {
if (k.code == 'Enter') // only if the key is "Enter"...
addTask(); // ...add a new task (using same handler as the button)
return false; // no propagation or default
}
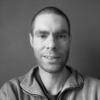
Tom Checkley
25,165 PointsI wanted to have a go at expanding Stevens answer (which was a massive help) So I came up with this that would work when enter was keyed on the edit task as well as the add task input. See what you think and let me know if there's a cleaner way of traversing.
var inputAddEdit = function(e) {
// find the key pressed
var key = e.code;
// check if it is Enter
if(key == 'Enter') {
// This is maybe where it is a little messy.
// We find the parentNode of the input from event.target
// We are traversing out of the li from the input with parentNode again.
// then finding it's id (i.e. incomplete-tasks or completed-tasks)
var grandParentId = e.target.parentNode.parentNode.id;
if(grandParentId == 'incomplete-tasks' || grandParentId == 'completed-tasks') {
// if it does match one of the edit input fields we do some more traversing to find the edit button
var editButton = e.target.parentNode.querySelector('button.edit');
// force a click to call the function
editButton.click();
} else {
// as the add task input grandparent has no id this will assume it is the addtask that has had enter pressed
// this would not work if there were other inputs in the document. an id would have to be added
addTask();
// bind enter key to new tasks
bindEnterKey();
}
}
};
var bindEnterKey = function() {
// find all text inputs
var textInputs = document.querySelectorAll('input[type=text]');
// cyple over text inputs to bind enter key use
for(var i = 0; i < textInputs.length; i++) {
textInputs[i].addEventListener('keypress', inputAddEdit);
}
};
// set click handler
addButton.addEventListener('click', addTask);
// call bindEnterKey for existing tasks
bindEnterKey();
//other remaining code....