Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial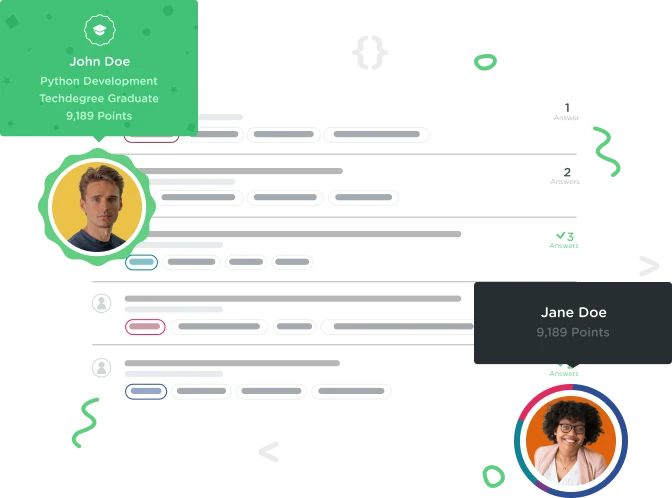

André Rybakken
459 PointsUse of "Continue"
Is the Continue statement really necessary in the infinite while-loop?
while True:
new_item = input("> ")
if new_item == 'DONE':
show_list()
break
add_to_list(new_item)
continue
1 Answer
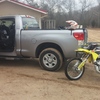
Ryan Ruscett
23,309 PointsHey,
You are right! The continue isn't necessary. Neither is the break. But it's good to know what they do. I only find em useful in very nested loop/if/elif type deals.
TIP! You need to ask yourself if a loop needs to be infinite. When I create a small server program. I will use While True, because I want the server to not be able to stop it. Although, in a program, you should leave a way out for the user. You should have a Boolean value like the one I used in this program for the exercise. So now I can turn it off whenever I type DONE - which is the second tip down below the code.
shopping_list = list() ; print("Pick up at the store?\n Enter 'DONE' to stop.")
Run = True
while Run:
new_item = raw_input("Item> ")
if new_item.upper() == 'DONE':
print("List has {} items.\n your list {}".format(len(shopping_list), shopping_list))
Run = False
shopping_list.append(new_item)
print("Added! List has {} items and here they are {}".format(len(shopping_list), shopping_list))
TIP - Think about if the user will actually type DONE DoNe or done. You still want it to exit properly. So add the .upper like I did above to do the check.
Does this answer your question? If so let me know, if not let me know how I can help clarify it more.
André Rybakken
459 PointsAndré Rybakken
459 PointsThanks for the swift response!
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsJust a note:
In Andre's copy of the code (sorry about the misspelling of your name) a continue or break is actually still necessary. If he removes it from his copy of the code the way it is, he'll end up with a shopping list that has 'DONE' as the last item, because add_to_list will run after the DONE conditional, adding that command to the list we're appending to. But continue isn't needed, you're right, because it's at the end of the loop already, so there's no code that we're trying to avoid running afterwards.
Also, in Ryan's copy of the code, if someone enters 'DONE' (or 'DonE', because they've accommodated for case-sensitive answers), five things will happen:
The list will print
Run will be changed to false, but the run condition won't be checked until the beginning of the next loop
'DONE' or 'DonE' will be appended to the end of the shopping list
Run will then be checked and found to be false, thus breaking the loop
The list will needlessly be printed again, this time with our 'DONE' command as the last item, as that print function is outside of our loop.
So you want something a little inbetween, to try and keep the code as dry as possible (non-repeatative) but still run well. Something like (and of course this might not be perfect, as I'm a beginner in python):
Because there's still code in the loop when we test for DONE, we still need some type of out. Using a boolean (the Run variable, booleans are True/False values) in the while loop instead of an infinite True loop means when we change Run to False, so we need to go back and run the while loop one more time to test the conditional, unlike the break, where we instantly break out of the loop. Just keep that in mind. And if you find yourself cutting and pasting a long print line like that, it probably means you can find a way of not doing that if you restructure the program. This is a good small exercise in refactoring code, and it was fun! Happy Coding!
Nicolas