Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial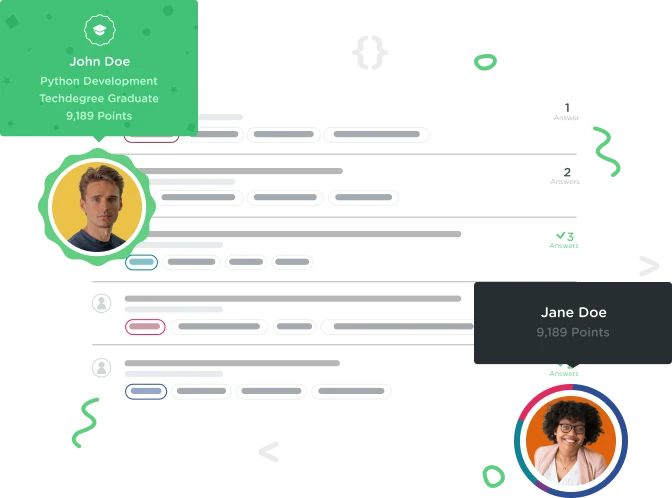

Sreeram Hareesh
2,014 PointsUse of "is" in python
Here is the code-
a="Done"
b="Done"
c=input("Enter Done :") #c takes the value "Done"
print(a is b)
print(a is c)
The first print statement prints True, whereas the second one prints False. Why is that happening?
1 Answer
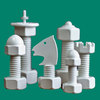
Steven Parker
229,732 PointsThe is operator is an identity test, unlike == which is an equality test. Your string literal referenced by both variables a and b (because Python optimized them to one object) is stored separately from the input string referenced by c. That's why "a is b" but not "a is c".
However, if you tried "a == c" you would find that to be True.
Sreeram Hareesh
2,014 PointsSreeram Hareesh
2,014 PointsAre there any particular names for the places where literals and the input strings are stored?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThe area where all objects are stored is call the "heap" (see docs). Variables names are stored in a namespace.
The namespace dictionary holds the name of the variable as the "keys" and the address of the object's location in the heap as the "value".
When an object is created (the right-side of the equals sign) if it is an immutable (unchangable) object then the heap of objects is checked to see if it is already present. This happens for strings and small numbers. If present, the variable name is pointed to the preexisting object.
The
id()
of an object is it's address in memory or "value" in the namespace dict. So if the id of two variables match, the object they reference is the same object in the heap.Sreeram Hareesh
2,014 PointsSreeram Hareesh
2,014 PointsThanks!