Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial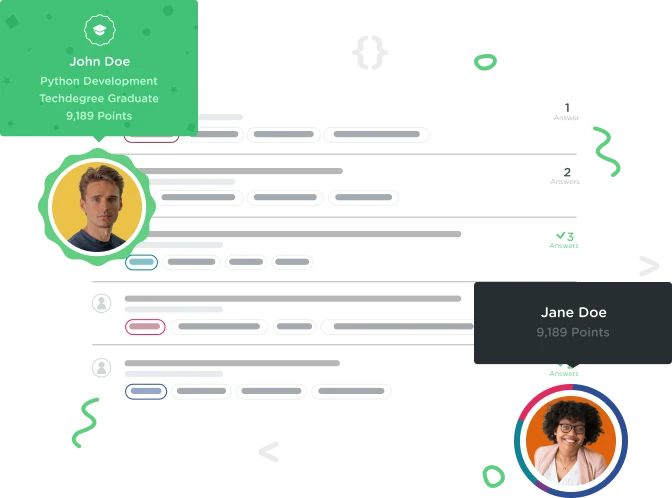

Augustine Makachemu
688 Pointsuse of unresolved identifier 'LocationManager()'
So my code in PermissionsController.swift has one issue and I keep getting the error... use of unresolved identifier 'LocationManager()'.
''' import UIKit import OAuth2
class PermissionsController: UIViewController {
let oauth = OAuth2ClientCredentials(settings: [
"client_id": "X4KP6ZK0ST-jF46KpAhxzg",
"client_secret": "m7TVywafskUun0OR0GeNbb9nP5sAUzbFGf8xVNv0bw9L4KPKg0O4lKiyYEETanI9",
"authorize_uri": "https://api.yelp.com/oauth2/token",
"secret_in_body": true,
"keychain": false
])
let locationManager = LocationManager()
var isAuthorizedForLocation: Bool
var isAuthenticatedWithToken: Bool
lazy var locationPermissionButton: UIButton = {
let title = self.isAuthorizedForLocation ? "Location Permissions Granted" : "Request Location Permissions"
let button = UIButton(type: .system)
let controlState = self.isAuthorizedForLocation ? UIControlState.disabled : UIControlState.normal
button.isEnabled = !self.isAuthorizedForLocation
button.setTitle(title, for: controlState)
button.addTarget(self, action: #selector(PermissionsController.requestLocationPermissions), for: .touchUpInside)
button.translatesAutoresizingMaskIntoConstraints = false
button.backgroundColor = UIColor(colorLiteralRed: 62/255.0, green: 71/255.0, blue: 79/255.0, alpha: 1.0)
button.setTitleColor(UIColor(colorLiteralRed: 178/255.0, green: 187/255.0, blue: 185/255.0, alpha: 1.0), for: .disabled)
button.setTitleColor(.white, for: .normal)
button.layer.cornerRadius = 5
button.clipsToBounds = true
return button
}()
lazy var oauthTokenButton: UIButton = {
let title = self.isAuthenticatedWithToken ? "OAuth Token Granted" : "Request OAuth Token"
let button = UIButton(type: .system)
let controlState = self.isAuthenticatedWithToken ? UIControlState.disabled : UIControlState.normal
button.isEnabled = !self.isAuthenticatedWithToken
button.setTitle(title, for: controlState)
button.addTarget(self, action: #selector(PermissionsController.requestOAuthToken), for: .touchUpInside)
button.translatesAutoresizingMaskIntoConstraints = false
button.backgroundColor = UIColor(colorLiteralRed: 62/255.0, green: 71/255.0, blue: 79/255.0, alpha: 1.0)
button.setTitleColor(UIColor(colorLiteralRed: 178/255.0, green: 187/255.0, blue: 185/255.0, alpha: 1.0), for: .disabled)
button.setTitleColor(.white, for: .normal)
button.layer.cornerRadius = 5
button.clipsToBounds = true
return button
}()
lazy var dismissButton: UIButton = {
let button = UIButton(type: .system)
button.setTitle("Dismiss", for: .normal)
button.tintColor = .white
button.addTarget(self, action: #selector(PermissionsController.dismissPermissions), for: .touchUpInside)
button.translatesAutoresizingMaskIntoConstraints = false
return button
}()
required init?(coder aDecoder: NSCoder) {
fatalError("init coder not implemented")
}
init(isAuthorizedForLocation authorized: Bool, isAuthenticatedWithToken authenticated: Bool) {
self.isAuthorizedForLocation = authorized
self.isAuthenticatedWithToken = authenticated
super.init(nibName: nil, bundle: nil)
}
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = UIColor(colorLiteralRed: 95/255.0, green: 207/255.0, blue: 128/255.0, alpha: 1.0)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
let stackView = UIStackView(arrangedSubviews: [locationPermissionButton, oauthTokenButton])
stackView.alignment = .center
stackView.axis = .vertical
stackView.distribution = .equalSpacing
stackView.spacing = 16.0
stackView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(stackView)
view.addSubview(dismissButton)
NSLayoutConstraint.activate([
locationPermissionButton.heightAnchor.constraint(equalToConstant: 64.0),
locationPermissionButton.leadingAnchor.constraint(equalTo: stackView.leadingAnchor),
locationPermissionButton.trailingAnchor.constraint(equalTo: stackView.trailingAnchor),
oauthTokenButton.heightAnchor.constraint(equalToConstant: 64.0),
oauthTokenButton.leadingAnchor.constraint(equalTo: stackView.leadingAnchor),
oauthTokenButton.trailingAnchor.constraint(equalTo: stackView.trailingAnchor),
stackView.centerYAnchor.constraint(equalTo: view.centerYAnchor),
stackView.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 32.0),
stackView.trailingAnchor.constraint(equalTo: view.trailingAnchor, constant: -32.0),
dismissButton.bottomAnchor.constraint(equalTo: view.bottomAnchor, constant: -16),
dismissButton.centerXAnchor.constraint(equalTo: view.centerXAnchor)
])
}
func requestLocationPermissions() {
do {
try locationManager.requestLocationAuthorization()
} catch LocationError.disallowedByUser {
// Show alert to user
} catch let error {
print("Location Authorization Error \(error.localizedDescription)")
}
}
func requestOAuthToken() {
oauth.authorize { authParams, error in
if let params = authParams {
guard let token = params["access_token"] as? String, let expiration
= params["expires_in"] as? TimeInterval else { return }
let account = YelpAccount(accessToken: token, expiration:
expiration, grantDate: Date())
do {
try? account.save()
self.oauthTokenButton.setTitle("OAuth Token Granted", for: .disabled)
self.oauthTokenButton.isEnabled = false
}
} else {
print ("Authorization was cancelled or went wong")
}
}
}
func dismissPermissions() {
dismiss(animated: true, completion: nil)
}
} '''