Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial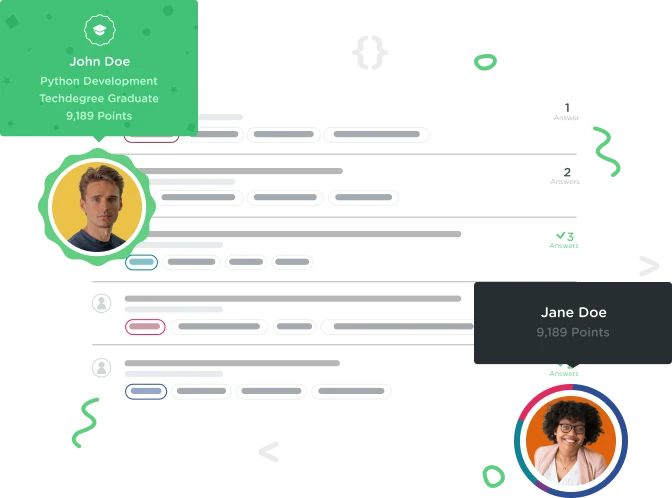

Sara U.
1,507 PointsUse of while int != False as opposed to while int == True. Why does one work when the other one does not?
I noticed on this challenge that when I use while x == True, no items will be added to my_list and therefore it will end up empty. Nonetheless, it does work if I write while x != False.
It does not only apply to this challenge, but doing simple while loops with a countdown from a given int, trying to get the code to print a message while the int is True.
What is the reason for this?
import random
def nchoices(iterable, n):
my_list = []
x = n
while x != False:
my_list.append(random.choice(iterable))
x -= 1
print(my_list)
nchoices('abcde', 5)
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Sara,
It has to do with how python treats boolean values. boolean is a subclass of int and that means the boolean values True and False are integers.
True
is the integer 1 and False
is the integer 0
So your while comparison is being done with integers.
The working condition x != False
is treated as x != 0
If x started at 5 we can see that this will be a true condition until x becomes 0, so it works out ok.
On the other hand, x == True
is treated as x == 1
. If x starts out as 5 we can see that it will be immediately false and never run.
You could convert your int to a boolean and I think it would work out. bool(x) == True
This will convert 5, for example, to the boolean True and then you have True == True
and that will be true and allow your while loop to run.
However, I don't recommend that you do any of the working ones here because it's less obvious what the code is doing when you're relying on this integer behavior of booleans.
Instead, you could just make your condition x
as Haider mentioned because it's more obvious what's happening.
Better yet, since you know how many times the loop is going to run, the value of n, a for loop is a better choice.
A for loop effectively has a built in counter so this eliminates 2 lines of your code. You don't have to initialize the counter before the loop and you don't have to decrement inside the loop.
Something like this can work too:
def nchoices(iterable, n):
my_list = []
for _ in range(n):
my_list.append(random.choice(iterable))
return my_list
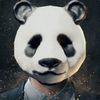
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi there,
int != False
would evaluate to True
because what you are doing here is checking if the value stored inside int
is literally equal to False
. Therefore, since the value inside int
is a number and not the Boolean value of False
, this would evaluate to True
as they are not the same thing. On the other hand, int == True
would evaluate to False
as once again, the value inside int
is a number, not a Boolean value.
Where you are going wrong here is instead of writing while x != False
or while x == True
, you should have just written while x
. This would cause the loop to continue as long as x evaluates to True
. Its quite tricky to explain but Kenneth does this perfectly so if you still don't understand, go back and re-watch the video ;).
Thanks,
Haider

Sara U.
1,507 PointsHi! Thanks so much, it makes total sense ^^
Sara U.
1,507 PointsSara U.
1,507 PointsThanks a lot for your comprehensive answer! Completely solved my question :)