Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial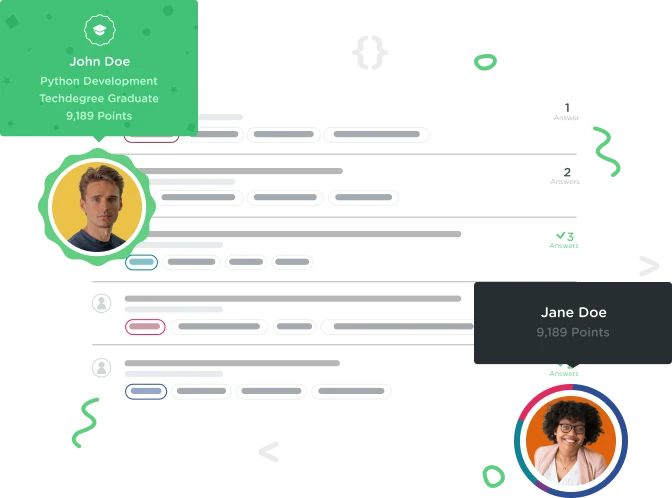

Mohammed Alkaff
1,563 PointsUse .remove() and/or del to remove the string, boolean, and list members of the_list.
Hi! Is it possible to solve the question with this code? I tried it in the workplace and it deletes all the items in the the_list. why cannt I preview it and why is it still wrong?
Thank you,
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0,the_list.pop(3))
for item in the_list:
while True:
items=item-1
try:
del the_list [items]
except:
break
8 Answers

Charles Ratkie
11,607 Pointsthe_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.pop(3)
the_list.insert(0, 1)
the_list.remove("a")
del the_list[3]
del the_list[3]
Keep in mind that when you remove "a", the list size changes. So 2 is now at index 0 etc. There are different ways to do it. :) Hope that helps
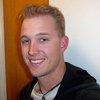
Richard Boag
5,547 PointsThere's a simpler way, puzzled me for a while too.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
the_list.insert(0, the_list.pop(3)) the_list.remove("a") the_list.remove(False) the_list.remove([1, 2, 3]) the_list.extend(range(1, 21))

David Diehr
16,457 PointsDylan's code almost works. However, for some reason it won't remove the last item in the list (figured out by using a simple print function). At first I assumed to had something to do with this item being a list but it doesn't. I tested switching the position of the list and the Boolean value and found that whichever was the last list item was not removed. I can't figure out why. But I do have a pretty easy work around, just straight up delete the last item. I added "del the_list[5]" the line before the for/loop and passed the code challenge. In fact, after figuring out this method succeeded I went back and found that you can just delete them all this way and don't even need the for/loop.
Not exactly quality learning but seeing as treehouse still has no mechanism for actually telling how you were supposed to approach the challenge or even a little mercy for those stuck on a challenge through dozens of failed attempts, I don't exactly feel guilty.
Side note, if anyone does know why Dylan's for/loop doesn't seem to iterate all the way through the list, I'd be interested to know.

David Rossing
810 PointsI too was really struggling with this one. After working on this for a while, I decided to take it in 2 parts. For the first part, I iterate through 'the_list' and find the indices that I want to remove. I add these indices to a new list called 'removal_index_list'. Then I iterate through the 'removal_index_list' and remove those indices from 'the_list'. There seems to be a bug that happens when you try to remove the item from 'the_list' while you're iterating on it, so seems like you have to do this one in 2 passes.
My solution (which passed):
tempitem = the_list.pop(3) the_list.insert(0, tempitem)
index = 0
removal_index_list = []
index = 0 for item in the_list: if type(item) is str or type(item) is bool or type(item) is list: removal_index_list.append(index) index = index + 1
removal_index_list.reverse()
for indx in removal_index_list: del the_list[indx]
print("final list") print(the_list)
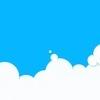
BHARTI PAREKH
5,085 Pointsmessy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
messy_list.pop(3) messy_list.insert(0 , 1) messy_list.remove("a") del messy_list[3] messy_list.remove([1, 2, 3])

ROBERT MACHOVO
7,034 Pointsi tried all of the above, but i am stl stuck
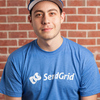
Dylan Shine
17,565 PointsThat is some funky stuff going on, try this:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
for item in the_list:
if type(item) is str or type(item) is bool or type(item) is list:
the_list.remove(item)
Haven't checked it but it should work

Jorge Dominguez
12,632 PointsDid not work. I would like to solve this challenge, I'm stuck too.

arpan rughani
1,457 Pointsadd this at the end del messy_list[3]

Richard Hanberry
762 PointsThis is what I got:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
the_list.insert(0, the_list.pop(3))
the_list.remove("a")
del the_list[3]
the_list.remove([1, 2, 3])
It worked after I figured out that del requires square brackets instead of parentheses :)

Richard Hanberry
762 PointsWhich is exactly the same as the above post