Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial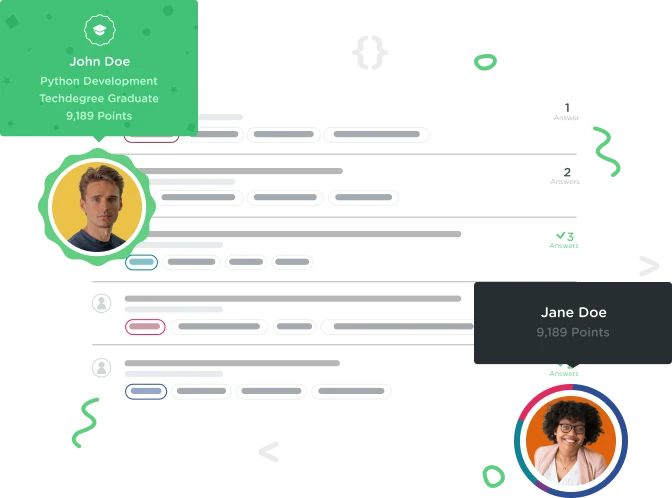
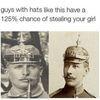
cody oberholtzer
1,988 PointsUse .remove() and/or del to remove the string, boolean, and list members of the_list.
Hello, not sure why this is not working. First it tells me Task 1 Error. Then in workspace it seems to work except for the list does not get removed. Thanks. Also not sure why pop does not use [3].
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0,the_list.pop(3))
for item in the_list:
if item != int:
print(item, " removed.\n")
the_list.remove(item)
else:
continue
1 Answer
William Li
Courses Plus Student 26,868 PointsHi there, Couple issues here. 1. if item != int:
is not the correct way of doing variable type checking in Python, type() build-in method is the tool for that job; 2. avoid deleting the list elements and thereby shrinking the list's length while traversing the list, doing so would almost always produce unexpected result in Python.
I believe this challenge only requires you to use either remove or del to delete those elements from the list one line at the time.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0,the_list.pop(3))
the_list.remove("a")
the_list.remove(False)
the_list.remove([1, 2, 3])
This would be a perfectly reasonable way of solving the part2 of the challenge. Normally, hardcoding like such and letting human doing all the work for the computer is considered bad programming, however, the intent of part2 is about giving you a chance to practice remove or del and be comfortable about using 'em to eliminate elements from a list. So that's fine in this case.
Later into the Python lecture, when you eventually learn about list comprehension, you can solve this challenge in one-line, like such.
the_list = [i for i in the_list if type(i) not in (str, bool, list)]
Cheers.
cody oberholtzer
1,988 Pointscody oberholtzer
1,988 PointsAwesome explanation thanks