Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial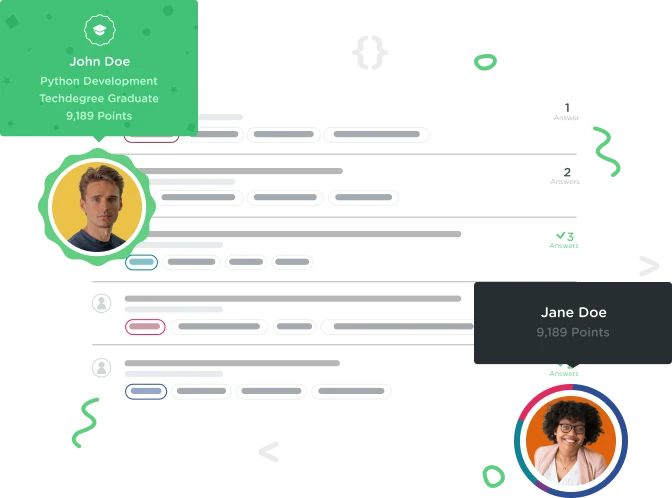

Harrison Cassedy
Java Web Development Techdegree Graduate 12,031 PointsUse the @Before to not repeat yourself.
I keep trying to solve the code but for some reason I keep getting the error " Please only initialize the Calculator object once in the setUp method that is annotated with @Before"
package com.example;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class CalculatorTest {
private Calculator calculator;
@Before
public void setUp() throws Exception {
Calculator calculator = new Calculator();
}
@Test
public void addingMultipleNumbersProducesResult() throws Exception {
Calculator calculator = new Calculator();
int answer = calculator.addNumbers(1 ,2, 3);
assertEquals(6, answer);
}
@Test
public void addingSingleNumberTotalsAppropriately() throws Exception {
Calculator calculator = new Calculator();
int answer = calculator.addNumbers(1);
assertEquals(1, answer);
}
}
package com.example;
public class Calculator {
public int addNumbers(int... numbers) {
int total = 0;
for (int number : numbers) {
total += number;
}
return total;
}
}