Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial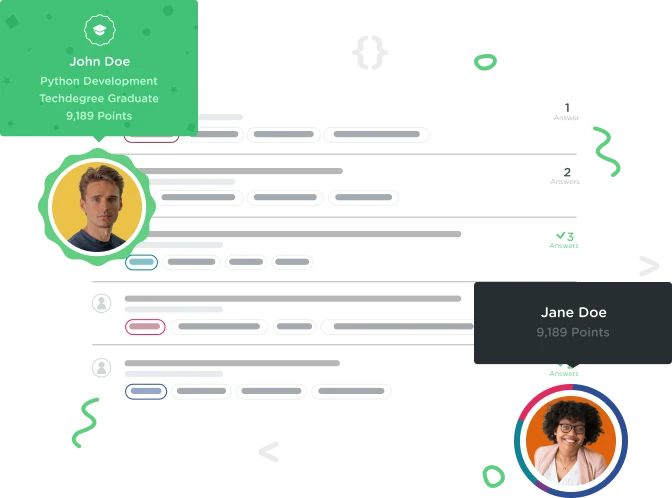

Gokul Nishanth
245 PointsUse the following keys to retrieve values from the dictionary: "title", "author", "price", "pubDate".
How to retrieve values from the dictionary? I have tried this method shown below: struct Book { let title: String let author: String let price: String? let pubDate: String?
init?(dict: [String : String]) {
return nil
}
}
func newBook(bookdict: [String : String]) -> Book? { guard let title = bookdict["title"], let author = bookdict["author"] else { return nil }
let price = bookdict["price"] let pubDate = bookdict["pubDate"]
return Book(title: title, author: author, price: price, pubDate: pubDate) }
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String : String]) {
return nil
}
}
func newBook(bookdict: [String : String]) -> Book? {
guard let title = bookdict["title"], let author = bookdict["author"] else {
return nil
}
let price = bookdict["price"]
let pubDate = bookdict["pubDate"]
return Book(title: title, author: author, price: price, pubDate: pubDate)
}
1 Answer
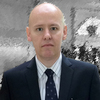
Nathan Tallack
22,159 PointsWhat you are wanting to do here is create an init function that will check to make sure the non optional properties are present in your dictionary and if they are not then return nil. We do that with a guard statement. It would look something like this.
init?(dict: [String: String]) {
guard let title = dict["title"], let author = dict["author"] else {
return nil
}
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
So we have the init? to show it an init that can fail, and we use a guard let (just like we do an if let) to pull the values for those non optional properties from the dictionary.
Note that unlike an if let the guard let will escape those values from its scope allowing them to be use further down in the init function where we are assigning them to our non optional properties.
Also note that we did not need to do this for the optional properties because if they are not there we will happily set them to nil because they are optional.
Swift is so pretty! :)