Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial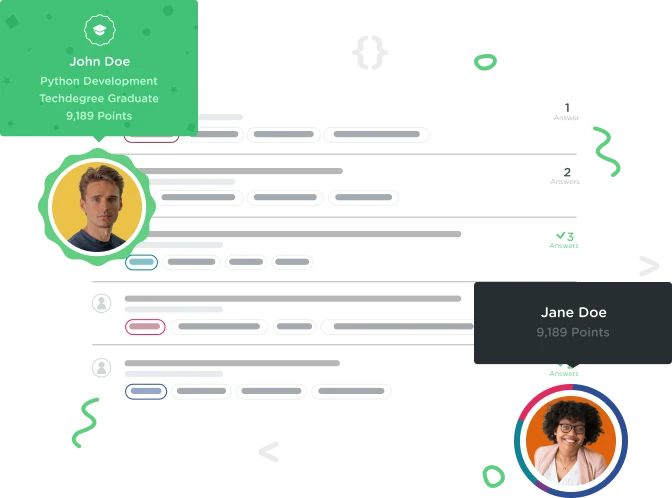

Neil Salazar
5,014 Pointsused an if else statement
so I spent some time trying to do this one, figured it out, I think, but am a little confused. Here's my solution:
function arrayCounter(array) {
if(typeof array === 'undefined') {
return 0;
}
if(typeof array === 'number') {
return 0;
}
if(typeof array === 'string') {
return 0;
}
else {
return array.length;
}
}
For a while I was trying to compare typeof array === 'array', until I realized/found out that I can't use typeof to compare an array. Can I? This let me pass, but if I were to put in a boolean, it returns undefined, but... oh wait I think I know why, because if typeof array === boolean, there is not array.length to return, thus it returns undefined, while passing an array will return array.length.
Is that right? Am I understanding this correctly?
3 Answers

Phillip Gibson
13,805 PointsThe way you solved it works as expected, however a few minor changes might improve it. Such as using an if-elseif-else
check instead of individual if
checks.
function arrayCounter(array) {
if(typeof array === 'undefined') {
return 0;
} else if(typeof array === 'number') {
return 0;
} else if(typeof array === 'string') {
return 0;
} else {
return array.length;
}
}
This actually won't matter much though, since if it passes any of the checks it will immediately return either way, so I doubt there is much of a performance difference.
Another alternative is to consolidate your type checking into one if
statement instead of multiple.
function arrayCounter(array) {
if(typeof array === 'undefined' || typeof array === 'number' || typeof array === 'string') {
return 0;
} else {
return array.length;
}
}
This is a bit more terse, and probably a more standard approach.
Finally, there is also another function not mentioned in that video called instanceof
which works similar to typeof
only it will compare actual types instead of casting the type to a string.
function arrayCounter(array) {
if(array instanceof Array) {
return array.length;
} else {
return 0;
}
}

Neil Salazar
5,014 PointsThanks for the response Phillip. I was looking for the last example of the operator instanceof! It appears much cleaner using the operator instanceof, to compare it to an array, I just didn't quite know how to use it. Much appreciated!

zvi raphael
3,225 Pointsthank you