Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial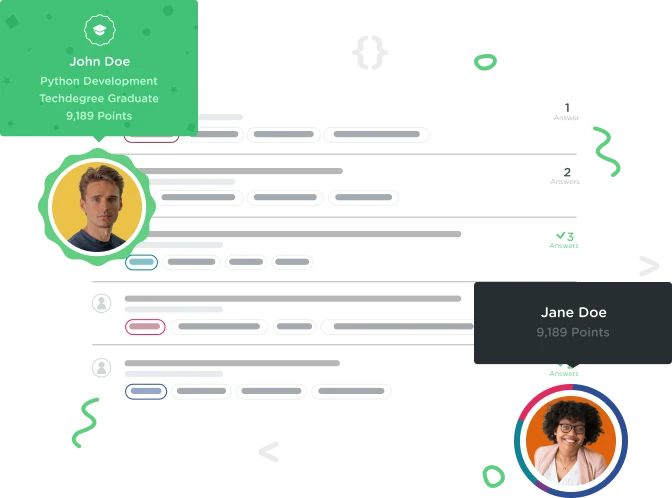
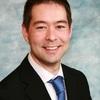
Mark Chesney
11,747 Pointsusefulness of <filter object> and <map object>
Hi. Out of curiosity, is there any usefulness to a map object, or a filter object, without having list()
around it? Examples:
a = [1, 2, 3, 4]
def get_square(x):
return x*x
map1 = map(get_square, a)
def is_even_num(x):
return x%2==0
filt1 = filter(is_even_num, a)
print(list(map1))
print(list(filt1))
The reason I ask is because when I type the following, only the dunders are returned:
dir(map1)
dir(filt1)
Hopefully this question doesn't take me down a rabbit hole of impractical curiosity. Would list(map(...))
and list(filter(...))` be typed out 90% of the time?
Thanks
1 Answer
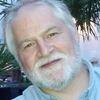
Jeff Muday
Treehouse Moderator 28,720 PointsI agree with you, 90% of the time, you're going to be immediately instantiating a list.
Guido van Rossum is not a fan of map(), filter(), and reduce(). He prefers comprehensions. He disliked reduce() so much that it was removed from Python 3. (Though it's retained in functools.reduce)
https://www.artima.com/weblogs/viewpost.jsp?thread=98196
https://www.python-course.eu/python3_lambda.php
My take on it is this-- by definition, an iterable is simply any object that can return an iterator. Iterables can range from complex data structures, to functional "infinite" structures like a cycles-- where a map() and filter() might be performed on sub-slices, to strings, to lists of integers, lists of floats, lists of strings, etc. The generic nature of the map() and filter() does not impose any kind of "opinionated" extra functionality ("opinionated" is a term often associated with forced static/dynamic typing). It is up to the programmer to instantiate their choice from the map() and filter() result.
I think Vincent Driesen's blog post is worth a read (I like how he presents iterators).
Mark Chesney
11,747 PointsMark Chesney
11,747 PointsThank you much Jeff! That second link you post is a great one!