Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial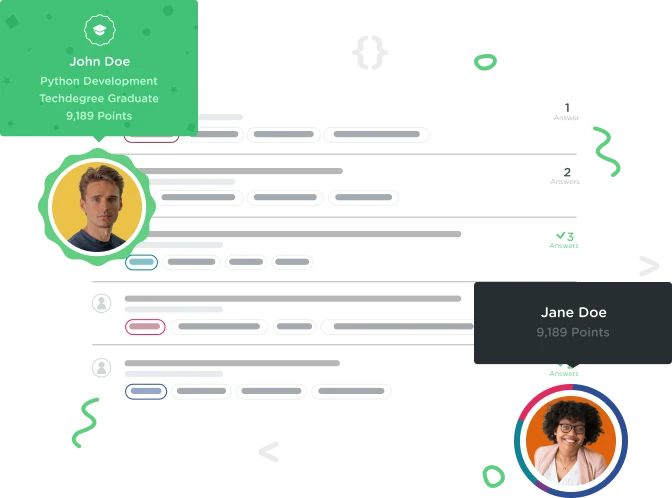

Dax Stucki
1,034 PointsUser author element still giving me "nullPointerException" error.
I'm at the last stage of the wrapping up challenge . I've fixed my code with the command line arguments as suggested but I'm still getting a "nullPointerException" error. Still not sure what else I can do.
thanks for any help provided
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description)
{
User mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription()
{
return mDescription;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName()
{
return mFirstName;
}
public String getLastName()
{
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic)
{
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User(args[0], args[1]);
// Add the author, title and description
ForumPost post = new ForumPost(author, "Done", "Woo Hoo");
forum.addPost(post);
}
}
3 Answers
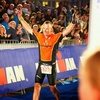
Steve Hunter
57,712 PointsHi Dax,
You are redeclaring your User
object in the ForumPost
constructor. This takes it out of scope of the class' instance variable:
public ForumPost(User author, String title, String description)
{
User mAuthor = author; // <- delete the class declaration User
mTitle = title;
mDescription = description;
}
You have declared mAuthor
to be a User
when you declared the member variable at the top of the class. Declaring it again means that the mAuthor
variable is redeclared in the constructor and is therefore limited in scope to just the constructor. That could easily cause Null Pointer Exceptions. Try deleting that unnecessary User
word in the constructor:
public ForumPost(User author, String title, String description) {
mAuthor = author; // use the mAuthor you've already created
mTitle = title;
mDescription = description;
}
Let me know how you get on.
Steve.

Dax Stucki
1,034 PointsTHANK YOU STEVE! Thanks so much, that works. I'm still a little confused about the "User mAuthor" variable that is declared at the start of ForumPost.java. is "User" the datatype? For some reason I can't wrap my head around this declaration.
thanks again. :]
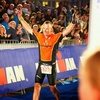
Steve Hunter
57,712 PointsHi Dax,
The member variable mAuthor
is an instance of the User
class. You've defined the User
class in your code; this is just one of those.
In this case, the member variable is part of the ForumPost
class; every ForumPost
has an mAuthor
which is of type User
. This instance/object is passed in as a parameter to the constructor of the ForumPost
class having been created in Example.java
with the line, User author = new User(args[0], args[1]);
So, ForumPost
has a User
-shaped hole in memory waiting to be filled. Then, when the ForumPost
instance is created, the User
object created in Example.java
is sent across to be used in the constructor for ForumPost
. In there, the ForumPost
instance stores the User
object in its member variable, mAuthor
. The member variable declaration creates the hole, the User
object instantiation in Example.java
creates a User
- the two are then merged, with the User
fitting the User
-shaped hole.
This is why you didn't need the extra User
keyword. The User
-shaped hole had already been created; that's the declaration of mAuthor
. The actual instance of User
was created in Example.java
and sent across for the constructor. Then, the two are put together with the instance filling the hole in memory that already existed in ForumPost
. When you had an extra User
declaration in there, an additional hole in memory was created with nothing to fill it - hence it was empty - a Null Pointer Exception.
In code:
// User constructor takes a first & last name
User author = new User(args[0], args[1]); // User created
// ForumPost constructor takes a User, title & description
ForumPost post = new ForumPost(author, "Done", "Woo Hoo"); // author object sent to ForumPost class
// Inside ForumPost, author object is used
public ForumPost(User author, String title, String description) {
mAuthor = author; // use the author you've created which this class is waiting for
...
That loosely covers what this was all about - I hope it made some sense to you!
Steve.

Dax Stucki
1,034 PointsThanks again. That makes sense now.
Awesome.
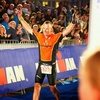
Steve Hunter
57,712 PointsNo problem!