Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial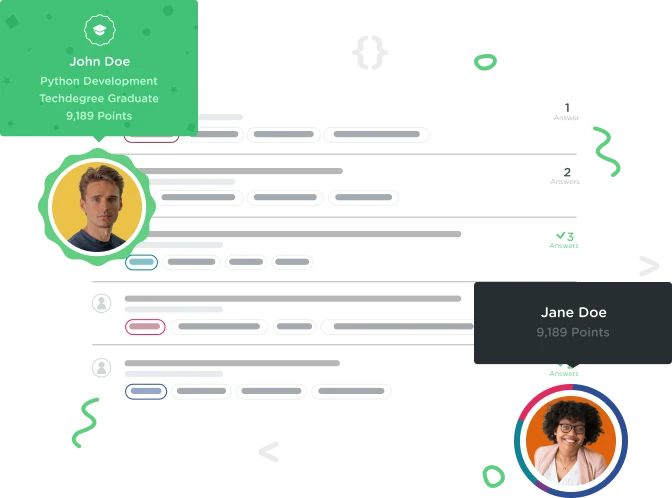

rubenlozano
1,096 PointsUser drawn triangle
I am trying to create a script that takes the user's mouse and draws a rectangle on canvas that is placed within the HTML, it also takes the width, height, area, and perimeter that is extracted from the location of the user's action, attached is my current code and i'm a bit confused, any pointers would be helpful
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="rect5.css">
<script src="rect5.js"></script>
</head>
<body onload="init()">
<form id="politics">
<fieldset id="candidates">
<legend>Canvas Form</legend>
<span>
<label for="width">Width</label>
<input type="number" class="width" id="wid" placeholder="Width"/>
<label for="height">Height</label>
<input type="number" class="height" id="hgt" placeholder="height"/>
</span>
</fieldset>
<br/>
<label for="area1">Area:</label>
<output id="area"></output>
<br/>
<label for="perimeter1">Perimeter:</label>
<output id="perim"></output>
<br/>
<button onclick="getarea()" type="button">Get Area</button>
</form>
<canvas id="drawing" width="500" height="500"></canvas><script src="rect5.js"></script>
</body>
</html>
//You can modify your getarea method from assignment 2 to add a call to draw a rectangle on the canvas from 0,0
function init() {
function drawarea(wid, hgt) {
"use strict";
var canvas = document.getElementById('drawing');
var context = canvas.getContext('2d');
context.clearRect(0, 0, canvas.width, canvas.height);
context.fillStyle = '#FF0000';
context.fillRect(0, 0, wid, hgt);}
function mouseControl() {
canvas.addEventListener("mousemove", function (e) {
findxy('move', e);
}, false);
canvas.addEventListener("mousedown", function (e) {
findxy('down', e);
}, false);
canvas.addEventListener("mouseup", function (e) {
findxy('up', e);
}, false);
canvas.addEventListener("mouseout", function (e) {
findxy('out', e);
}, false);}
drawarea(100,100);
}
function getarea() {
var wid = document.getElementById("wid").value;
var hgt = document.getElementById("hgt").value;
var area = wid * hgt;
var perim = ( Number(wid) + Number(hgt) ) * 2;
document.getElementById('area').innerHTML = area;
document.getElementById('perim').innerHTML = perim;
}

Jason Anello
Courses Plus Student 94,610 PointsIt might be easier to get help on this if you could explain what steps the user is supposed to take when interacting with this.

rubenlozano
1,096 PointsGood point Anello,
This is my first post so I appreciate the guidance :)
First part is the left mouse click will begin drawing the rectangle, the user will drag out the rectangle, and releasing the mouse will establish the final size of the rectangle. The current width and height of the rectangle should be fed back to the user while the mouse button is depressed. There are several ways to do this, including filling in the form values (which I tried to do with my perimeter function). If you provide feedback to the user through a popup or other mechanism, you may defer filling in the form until the user has released the mouse (I don’t see a point in a popup, but maybe it is an option?). Conversely, if the user fills out the form and presses submit, draw a rectangle on the canvas from 0,0 to the extent provided by the user (This is a confusing part because I already have the function in the code that can calculate the perimeter and area, but I need to transfer that to the canvas, which is another part that has me confused). The canvas at the very least needs to be 500 x 500 and I need to ensure my form input does not accept sizes bigger than your canvas, or less than or equal to zero. The line and file of the rectangle is up to me, but obviously that isn’t the biggest concert :/
1 Answer
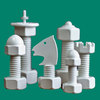
Steven Parker
230,274 PointsDid you ever resolve your issues? I just happened to find this post and I see that mouseControl is defined but never called, but canvas is never defined in it.
Also, a function named "findxy" is involved in all the mouse actions but it is never defined.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointsfixed code formatting