Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial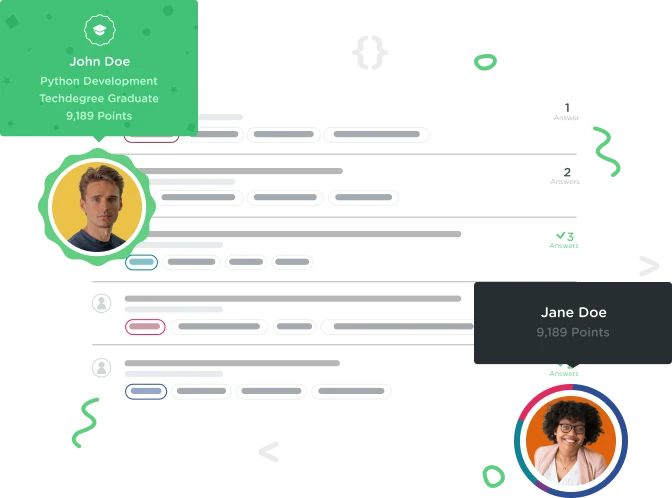
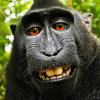
Juan de Elena O'Shea
4,757 Pointsuser input into functions on JavaScript
Hello all!
While struggling with this challenge, I thought that it would be better to pass the arguments into the function as a user (on the web page) instead of through the code, as shown by Dave (console.log(getRandomNumber(1, 100));) so I fiddled through to something like this:
function getRandomNumber (lower, upper) {
return random = Math.floor(Math.random() * (upper - lower +1)) + lower;
}
// console test:
console.log(getRandomNumber(1, 100));
// real thing:
var upper = prompt ("Input highest number in the range you want to use.");
var lower = prompt ("Input lowest number in the range you want to use.");
alert (getRandomNumber(lower, upper));
But to my surprise (well, not really, I am not surprised when my code does not work as expected :) ) it works in a very weird way, for example, when passing 20 and 10 as arguments, it spits out results like 310, 210, etc...
Can someone kindly guide me through what's going on here?
Many thanks for your help.
Kind regards,
Juan
2 Answers

Gunhoo Yoon
5,027 PointsAnything coming from prompt is treated like a string and JavaScript tries to concatenate with + operator when it sees a string in one of operands. So to fix this you need to cast your variable to number type. You can either use parseInt() Number() I recommend you use parseInt().
var upper = prompt ("Input highest number in the range you want to use.");
var lower = prompt ("Input lowest number in the range you want to use.");
// Convert value before passing into getRandomNumber function.
// When user supply invalid input it will produce NaN.
// Second argument 10 means always convert it to decimal.
upper = pasreInt(upper, 10)
lower = parseInt(lower, 10)
alert (getRandomNumber(lower, upper));
//P.S: You can also do parseInt(prompt(message), 10)
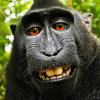
Juan de Elena O'Shea
4,757 PointsOh!!!! Cool!
Many thanks for your help Gunhoo. And very clear explanation.
Kind regards,
Juan

Gunhoo Yoon
5,027 Pointsyour welcome let me know if it doesn't work.