Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial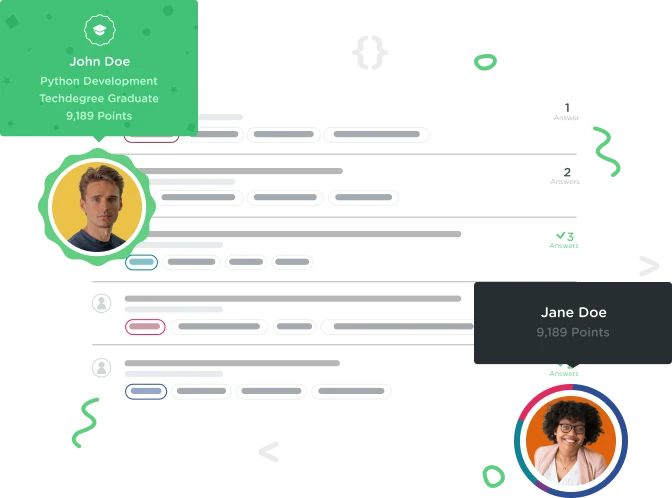

Evan Osczepinski
5,189 PointsUser-defined Functions help
The challenge is giving me some trouble. this is step 2 of 3. This is what I have so far. I am not sure how to keep the running total for this number sequence even after watch the video and the video after this challenge. Help please and thank you!
Write the code inside this mimic_array_sum() function. That code should add up all individual numbers in the array and return the sum. You’ll need to use a foreach command to loop through the argument array, a working variable to keep the running total, and a return command to send the sum back to the main code.
<?php
function mimic_array_sum ($array) {
$sum=
foreach($array as $number) {
$sum = $sum + ;
}
return=$sum;
}
$palindromic_primes = array(11, 757, 16361);
?>
4 Answers
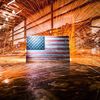
Andrew Shook
31,709 PointsYour have a few syntax errors. First, you need to either declare a value for $sum ( i.e. $sum = 0) or you need to remove the equals sign. Second, you need a semi-colon at the end of that line. Next, you either need to add a value after the + or rearrange that line of code. There is a shorthand in php for adding and setting a variable simultaneously, +=. The value you need to add to $sum is $number. If $array is a list of numbers then $number is each number in the list as foreach loops through the list. So you can write $sum = $sum + $number; or you could use the shorthand and write $sum += $number;. Finally, you have a syntax error on the return line. You need to remove the equals sign. You should only use the equals sign when you are assigning a value to a variable.
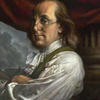
Jeff Busch
19,287 PointsHi Evan,
You're pretty close. First you need to make sure the variable $sum is set to zero. Second, you need to add the working variable $number to $sum.
Jeff
<?php
function mimic_array_sum($array)
{
$sum = 0;
foreach($array as $number) {
$sum = $sum + $number;
}
return $sum;
}
$palindromic_primes = array(11, 757, 16361);
$sum = mimic_array_sum($palindromic_primes);
echo $sum;
?>
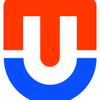
anguswhiston
17,225 PointsOne thing that I'm getting confused on is what needs to be labeled as what. Does the initial line
mimic_array_sum($array) always need to have it as $array? Or is that just for show and it could be anything?
Once we've written our own function and well call $sum = mimic_array_sum does that NEED to be $sum? Or can it be anything, I know we've got $sum in the function but am I right in thinking the whole point is they don't 'know' about each other?
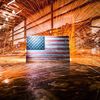
Andrew Shook
31,709 PointsIn the real word you could write the function like this:
<?php
function mimic_array_sum($x){
$z = 0;
foreach($x as $y) {
$z = $z + $y;
}
return $z;
}
$a = array(11, 757, 16361);
$whatever = mimic_array_sum($a);
echo $whatever;
?>
It doesn't really matter what variable name you give a parameter ( $x in my example or $array in the original function) because parameter variables are just a place to temporarily store and a way to access information that you are passing into a function. All that the PHP engine knows is that when you use the function mimic_array_sum and you pass in $a, it should replace every instance of $x within the function with $a. It also doesn't matter what you call a variable inside a function since the variable only exists inside that function. Once the function ends all those variables are deleted from memory. You can also use the same variable name inside and outside a function since PHP knows there is a difference between the two. This is called scoping, and works something like this. All variables declared outside of a function have a global scope meaning everything in the PHP script can see them but may or may not be able to change the value of the variable. All variable declared as a parameter or inside a function belong to that function. Nothing outside that function can changes those variables. This mean you could write the example above like this:
<?php
function mimic_array_sum($x){
$a = 0;
foreach($x as $y) {
$a = $a + $y;
}
return $a;
}
$a = array(11, 757, 16361);
$whatever = mimic_array_sum($a);
echo $whatever;
?>
And PHP ( and all programming languages I can think of ) will not erase the array outside the function and give it a value of zero when the function it called. Because for PHP Global $a is different from mimic_array_sum $a.
Now, on here on treehouse everything I just said may or may not apply. It is because they need a way to check your work. I know that on some code challenges they do a string match, meaning they compare what you wrote to what the answer should be. If you forgot a period inside of a string then your answer is wrong. On others, like the example above, they check the value of the variable , $sum, against their master answer. Hope this answered your question.

Evan Osczepinski
5,189 PointsThank you Jeff and Andrew. I am having trouble with functions. They just aren't clicking with me. These responses have definitely helped a lot!