Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial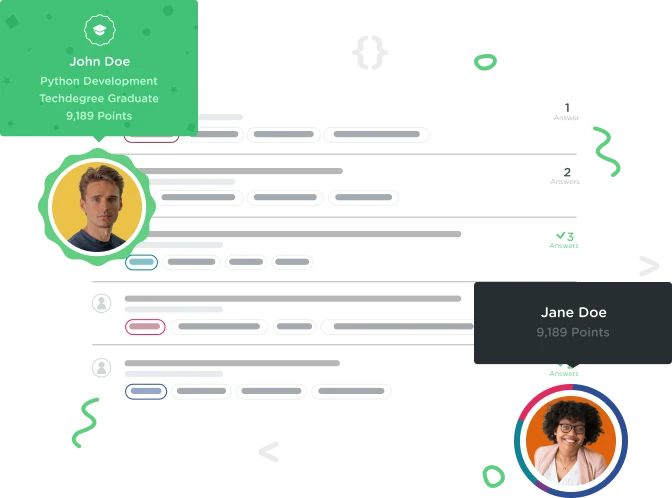

Marcos Cabrini Riani dos Reis
12,253 PointsUser.findOne(...).exc is not a function
Using this code below, when I try to log in this error message appears "User.findOne(...).exc is not a function"
index.js code
=====================================
var express = require('express');
var router = express.Router();
var User = require('../models/user');
// GET / profile
router.get('/profile', function (req, res, next) {
if (!req.session.userId) {
var err = new Error('You are not authorized to view this page.');
err.status = 403;
return next(err);
}
User.findById(req.session.userId)
.exec(function (error, user) {
if (error) {
return next(error);
} else {
return res.render('profile', { title: 'Profile', name: user.name, favorite: user.favoriteBook });
}
});
});
// GET / logins
router.get('/login', function (req, res, next) {
return res.render('login', { title: 'Log In' });
});
// POST /logins
router.post('/login', function (req, res, next) {
if (req.body.email && req.body.password) {
User.authenticate(req.body.email, req.body.password, function (error, user) {
if (error || !user) {
var err = new Error('Wrong email or password.');
err.status = 401;
return next(err);
} else {
req.session.userId = user._id;
return res.redirect('/profile');
}
});
} else {
var err = new Error('Email and password are required.');
err.status = 401;
return next(err);
}
});
// GET / Register
router.get('/register', function (req, res, next) {
return res.render('register', { title: 'Sign Up' });
});
// POST / register
router.post('/register', function (req, res, next) {
if (req.body.email &&
req.body.name &&
req.body.favoriteBook &&
req.body.password &&
req.body.confirmPassword) {
// confirm that user typed same password twice
if (req.body.password !== req.body.confirmPassword) {
var err = new Error('Passwords do not match.');
err.status = 400;
return next(err);
}
// creat object with form input
var userData = {
email: req.body.email,
name: req.body.name,
favoriteBook: req.body.favoriteBook,
password: req.body.password,
};
// use schema's `create` method to insert document into mongo
User.create(userData, function (error, user) {
if (error) {
return next(error);
} else {
req.session.userId = user._id;
return res.redirect('/profile');
}
});
} else {
var err = new Error('All fields required.');
err.status = 400;
return next(err);
}
});
// GET /
router.get('/', function (req, res, next) {
return res.render('index', { title: 'Home' });
});
// GET /about
router.get('/about', function (req, res, next) {
return res.render('about', { title: 'About' });
});
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});
module.exports = router;```
1 Answer

Seth Kroger
56,413 PointsFrom the text of your question it looks like you are misspelling exec() somewhere in your code. It's not present in the code you posted so it's probably in another file.
Gari Merrifield
9,597 PointsGari Merrifield
9,597 Pointsthis is near impossible to read without the code being in the code blocks properly. We can't tell what may be comments and what may be code. Please edit your question for us, if you still need help. See the "Markdown Cheatsheet" link on this page if you need help with the code blocks.