Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial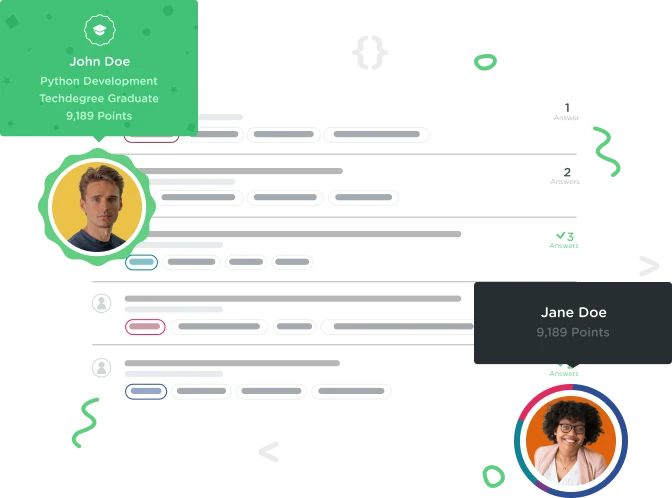

Max Larsen
3,024 PointsUsername is not replacing the %1$s
My code for the story class looks like this:
public class Story { private Page[] mPages;
public Story() {
mPages = new Page[7];
mPages[0] = new Page(
R.drawable.page0,
"On your return trip from studying Saturn's rings, you hear a distress signal that seems to be coming from the surface of Mars. It's strange because there hasn't been a colony there in years. Even stranger, it's calling you by name: \"Help me, %1$s, you're my only hope.\"",
new Choice("Stop and investigate", 1),
new Choice("Continue home to Earth", 2));
mPages[1] = new Page(
R.drawable.page1,
"You deftly land your ship near where the distress signal originated. You didn't notice anything strange on your fly-by, but there is a cave in front of you. Behind you is an abandoned rover from the early 21st century.",
new Choice("Explore the cave", 3),
new Choice("Explore the rover", 4));
mPages[2] = new Page(
R.drawable.page2,
"You continue your course to Earth. Two days later, you receive a transmission from HQ saying that they have detected some sort of anomaly on the surface of Mars near an abandoned rover. They ask you to investigate, but ultimately the decision is yours because your mission has already run much longer than planned and supplies are low.",
new Choice("Head back to Mars to investigate", 4),
new Choice("Continue home to Earth", 6));
mPages[3] = new Page(
R.drawable.page3,
"Your EVA suit is equipped with a headlamp, which you use to navigate the cave. After searching for a while your oxygen levels are starting to get pretty low. You know you should go refill your tank, but there's a very faint light up ahead.",
new Choice("Refill at ship and explore the rover", 4),
new Choice("Continue towards the faint light", 5));
mPages[4] = new Page(
R.drawable.page4,
"The rover is covered in dust and most of the solar panels are broken. But you are quite surprised to see the on-board system booted up and running. In fact, there is a message on the screen: \"%1$s, come to 28.543436, -81.369031.\" Those coordinates aren't far, but you don't know if your oxygen will last there and back.",
new Choice("Explore the coordinates", 5),
new Choice("Return to Earth", 6));
mPages[5] = new Page(
R.drawable.page5,
"After a long walk slightly uphill, you end up at the top of a small crater. You look around, and are overjoyed to see your favorite android, %1$s-S1124. It had been lost on a previous mission to Mars! You take it back to your ship and fly back to Earth.");
mPages[6] = new Page(
R.drawable.page6,
"You arrive home on Earth. While your mission was a success, you forever wonder what was sending that signal. Perhaps a future mission will be able to investigate...");
}
public Page getPage(int pageNumber) {
return mPages[pageNumber];
}
}
And the code for the loadPage function looks like this:
private void loadPage() { Page page = mStory.getPage(0);
Drawable drawable = getResources().getDrawable(page.getImageId());
mImageView.setImageDrawable(drawable);
String pageText = page.getText();
// Add the name if placeholder included. Won't add if not placeholder
pageText = String.format(pageText, mName);
mTextView.setText(page.getText());
mChoice1.setText(page.getChoice1().getText());
mChoice2.setText(page.getChoice2().getText());
}
Thank you so much!
4 Answers
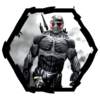
channonhall
12,247 Pointshey I don't know what is the problem but I just want to tell you that you could download the file for this. or copy and paste this code :
''' /**
-
Created by Channon hall on 1/14/16. */ public class Story { private Page[] mPages;
public Story() { mPages = new Page[7];
mPages[0] = new Page( R.drawable.page0, "On your return trip from studying Saturn's rings, you hear a distress signal that seems to be coming from the surface of Mars. It's strange because there hasn't been a colony there in years. Even stranger, it's calling you by name: \"Help me, %1$s, you're my only hope.\"", new Choice("Stop and investigate", 1), new Choice("Continue home to Earth", 2)); mPages[1] = new Page( R.drawable.page1, "You deftly land your ship near where the distress signal originated. You didn't notice anything strange on your fly-by, but there is a cave in front of you. Behind you is an abandoned rover from the early 21st century.", new Choice("Explore the cave", 3), new Choice("Explore the rover", 4)); mPages[2] = new Page( R.drawable.page2, "You continue your course to Earth. Two days later, you receive a transmission from HQ saying that they have detected some sort of anomaly on the surface of Mars near an abandoned rover. They ask you to investigate, but ultimately the decision is yours because your mission has already run much longer than planned and supplies are low.", new Choice("Head back to Mars to investigate", 4), new Choice("Continue home to Earth", 6)); mPages[3] = new Page( R.drawable.page3, "Your EVA suit is equipped with a headlamp, which you use to navigate the cave. After searching for a while your oxygen levels are starting to get pretty low. You know you should go refill your tank, but there's a very faint light up ahead.", new Choice("Refill at ship and explore the rover", 4), new Choice("Continue towards the faint light", 5)); mPages[4] = new Page( R.drawable.page4, "The rover is covered in dust and most of the solar panels are broken. But you are quite surprised to see the on-board system booted up and running. In fact, there is a message on the screen: \"%1$s, come to 28.543436, -81.369031.\" Those coordinates aren't far, but you don't know if your oxygen will last there and back.", new Choice("Explore the coordinates", 5), new Choice("Return to Earth", 6)); mPages[5] = new Page( R.drawable.page5, "After a long walk slightly uphill, you end up at the top of a small crater. You look around, and are overjoyed to see your favorite android, %1$s-S1124. It had been lost on a previous mission to Mars! You take it back to your ship and fly back to Earth."); mPages[6] = new Page( R.drawable.page6, "You arrive home on Earth. While your mission was a success, you forever wonder what was sending that signal. Perhaps a future mission will be able to investigate...");
}
public Page getPage(int pageNumber) { return mPages[pageNumber]; } } ''' and figure out what is the problem
hope it helps :)
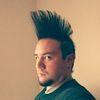
Ben Morse
6,068 PointsHey, if you are getting a null instead of your name in the placeholder, then you have to remove the String form String mName
String mName = intent.getStringExtra(getString(R.string.key_name));
to
mName = intent.getStringExtra(getString(R.string.key_name));
reason being is that you already declared it in your member variable section
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private String mName1;
Because I notice that my IDE was telling me Hey! you haven't used mName because it was grayed out....I'm like "Yes i have, right there!" Then i thought okay...i'll just remove the String data and bam! it wasn't grayed out anymore.
Best of luck friend! Hope that helped :)

Juan Ferr
13,220 PointsThat was because you were declaring it again and overriding it's content

Tal Dabush
1,332 PointsThank you! helped alot.
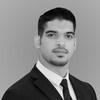
Laith Mohammad
6,569 PointsU r the man!
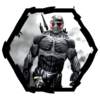
channonhall
12,247 Pointsforgot to add this too
''' Drawable drawable = getResources().getDrawable(mCurrentPage.getImageId()); mImageView.setImageDrawable(drawable);
String pageText = mCurrentPage.getText();
// Add the name if placeholder included. Won't add if no placeholder
pageText = String.format(pageText, mName);
mTextView.setText(pageText);
if (mCurrentPage.isFinal()) {
mChoice1.setVisibility(View.INVISIBLE);
mChoice2.setText("PLAY AGAIN");
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
else {
mChoice1.setText(mCurrentPage.getChoice1().getText());
mChoice2.setText(mCurrentPage.getChoice2().getText());
mChoice1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getChoice1().getNextPage();
loadPage(nextPage);
}
});
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getChoice2().getNextPage();
loadPage(nextPage);
}
});
}
}
} '''
And if its not working please tell me asap

Andre Nuzzolillo
1,681 Pointsprivate void loadPage(){
Page page = mStory.getPage(0);
Drawable drawable = getResources().getDrawable(page.getImageId());
mImageView.setImageDrawable(drawable);
String pageText = page.getText();
// This will add the name if place holder included (:
pageText = String.format(pageText, mName);
mTextView.setText(pageText);
mChoice1.setText(page.getChoice1().getText());
mChoice2.setText(page.getChoice2().getText());
}
You just need to set the text of "mTextView" with "pageText" variable and it should work.
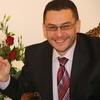
Mazen Itani
1,614 PointsGood work Andre, it worked