Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial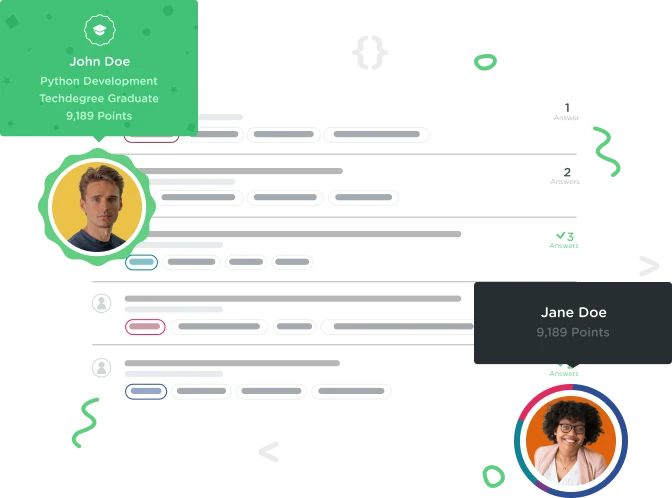

Riley Bolen
26,711 PointsUsing a custom layout as a dialog
I am trying to have a custom layout that I have created open as a dialog when a button on my app is pressed. I already figured out how to have a simple dialog with a title, message and positive button display when the button is pressed, but now I have been trying to change it to the custom layout and the app crashes when the button is pressed. Here are the files. The xml layout is just a rough draft, but the basic components are there.
NewTaskDialogFragment.java:
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.DialogFragment;
import android.content.Context;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
public class NewTaskDialogFragment extends DialogFragment implements
android.view.View.OnClickListener {
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context);
LayoutInflater inflater = LayoutInflater.from(context);
View dialogView= inflater.inflate(R.layout.new_task_dialog, null);
builder.setView(dialogView);
builder.show();
return builder.create();
}
@Override
public void onClick(View view) {
}
}
new_task_dialog.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#919191">
<EditText
android:layout_width="match_parent"
android:layout_height="100dp"
android:inputType="textMultiLine"
android:ems="10"
android:id="@+id/editText"
android:background="#ffffff"
android:layout_marginTop="20dp"
android:layout_marginBottom="20dp"
android:hint="What do you need to get done this week?"
android:gravity="top"
/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="2">
<Spinner
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/spinner2"
android:layout_weight="1"
/>
<Spinner
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/spinner"
android:layout_weight="1"/>
</LinearLayout>
</LinearLayout>
Relevant parts of MainActivity.java (showTaskOnClick is called in OnCreate):
private void showTaskOnClick(){
Button addTaskButton = (Button) findViewById(R.id.addTaskButton);
addTaskButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
showTaskBox();
}
});
}
private void showTaskBox(){
NewTaskDialogFragment dialog = new NewTaskDialogFragment();
dialog.show(getFragmentManager(), "");
}
Thanks in advance for the help!
1 Answer

Seth Kroger
56,414 Pointshttps://developer.android.com/reference/android/app/AlertDialog.Builder.html says to use create() OR show() but not both since they do the same thing. show() just takes the extra step of displaying the dialog. Since the DialogFragment should take care of showing it, use should use create().
Riley Bolen
26,711 PointsRiley Bolen
26,711 PointsThanks, that did it!